HTML generation is an important part of traditional web applications where the server builds HTML dynamically based on what the user has input into the application.
Old, dangerous way
In the olden days, many web applications constructed HTML by simply concatenating strings. Here is an example of a "legacy" PHP application that returns HTML containing a search term taken from user input:
echo "<p> Search results for search: " . $_GET('search') ."</p> "
If the user would go to the URL address https://www.example.com/?haku=kissa, the application would return the HTML to the browser:
<p>Search results for: cat</p>
The main reason why this is a terribly poor and dangerous way to build dynamic HTML is that the structure of HTML and the data displayed in HTML can easily get mixed up. What if the user had gone to this URL address? https://www.example.com/?haku=<script>alert('XSS')</script>
Then the application would return HTML with JavaScript code that was not intended to be there.
<p>Search results for:<script>
alert('XSS')
</script></p>
This phenomenon is called an XSS vulnerability, from which you can learn more in the XSS course.
Using Flask with HTML templates is quite straightforward and requires only a few basic steps.
Correct way: Templates
The correct way to build dynamic HTML is to use a template library such as Jinja2, which Flask natively uses.
Using Jinja2 templates in Flask applications is relatively easy. Here is a brief description of how you can use Jinja2 templates in Flask:
- Install Jinja2 using Flask: Jinja2 is usually installed with Flask, but make sure you have the latest version. You can check it with the command "pip show Flask".
- Create Jinja2 templates in Flask application: Create a template (templates) using Jinja2 syntax in the "templates" folder of the Flask application. You can use Jinja2 template language in the template, which provides various functionalities such as loops, conditions, and variables.
- Pass information to the template in Flask application: You can pass data from a Flask application to a Jinja2 template using Flask's "render_template" function. This function creates an HTML page that uses the Jinja2 template and passes data to it.
- Show the template in the browser: When the Flask application receives a request, it calls the view function, which uses the render_template function to send an HTML page generated using the Jinja2 template.
Simple Example
main.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html', name='World')
if __name__ == '__main__':
app.run(debug=True)
templates/index.html
<!DOCTYPE html><html><head><title>Flask & Jinja2 Example</title></head><body><h1> Hello, {{ name }}!</h1></body></html>
Practice
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
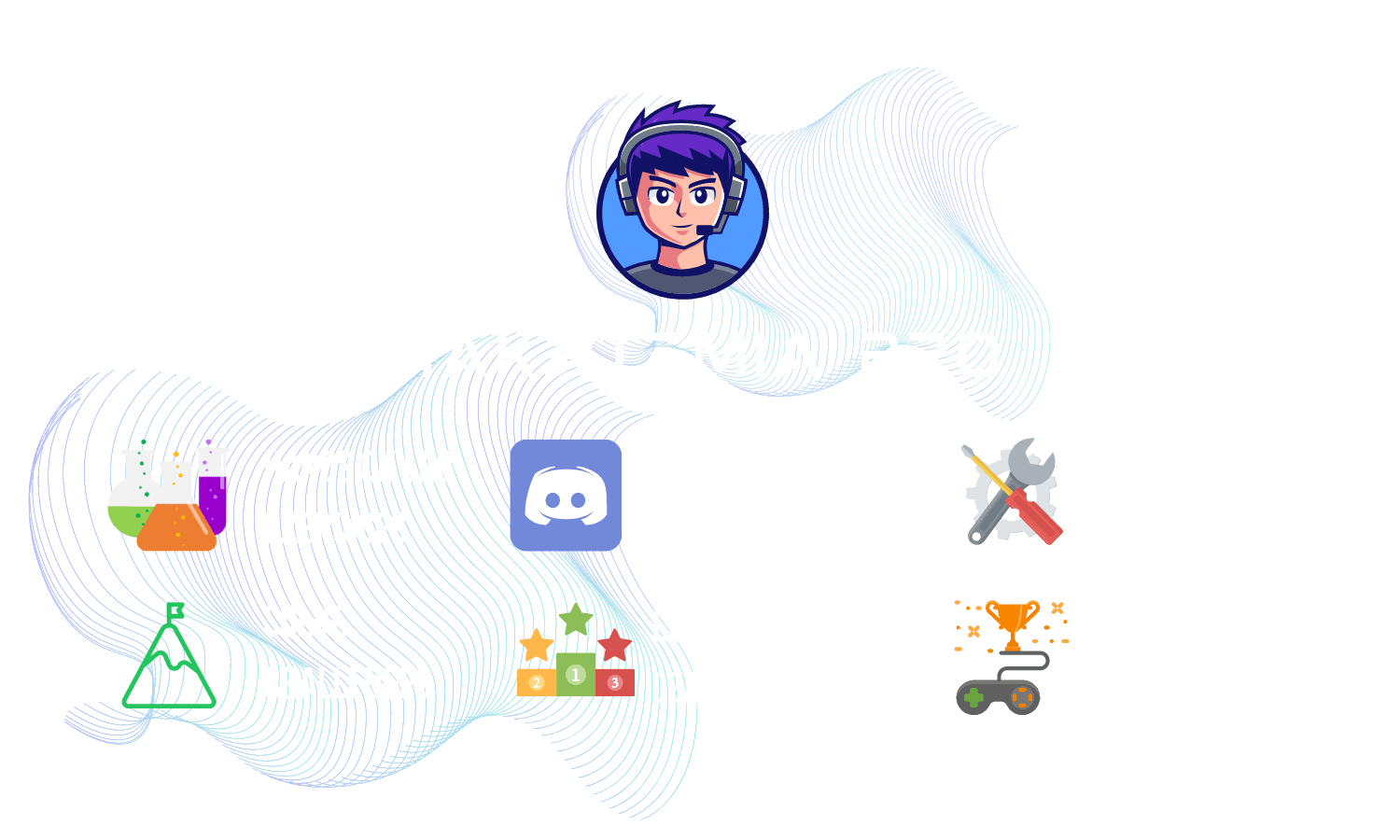
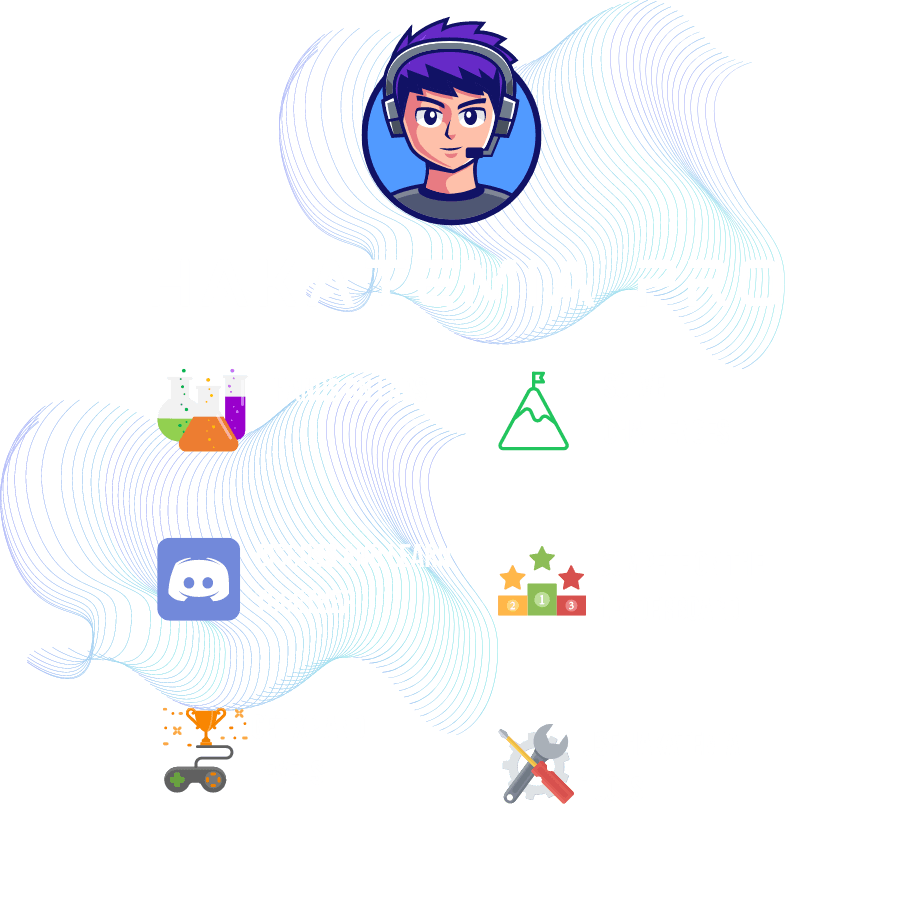
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.