Conditional statements are used in programming to perform different actions based on whether certain conditions are met or not. This allows control and decision making in the execution of the program. JavaScript has several different types of conditional statements, but the most common ones are if statements and switch statements.
if statements
if statements are used to check if a certain condition is true and to execute specific code accordingly. For example, you can use if statements to check if a variable is equal to another:
var x = 5;
var y = 10;
if (x == y) {
console.log("Variables are equal");
} else {
console.log("Variables are not equal");
}
This checks if the variables "x" and "y" are equal. If they are, the code executes the first sentence, otherwise the second sentence.
Switch statements
Switch statements are used to check the value of a variable and execute code based on it. For example, you can use switch statements to check which day of the week it is:
var day = 4;
switch(day) {
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
case 4:
console.log("Thursday");
break;
case 5:
console.log("Friday");
break;
case 6:
console.log("Saturday");
break;
case 7:
console.log("Sunday");
break;
default:
console.log("Invalid value");
}
This checks which day of the week it is using the variable "paiva". Different case statements are used in the switch statement to check which day corresponds to the given value. If the day corresponds to a case statement, the code executes that statement and then stops. If no case statement corresponds to the given value, the default statement is executed.
Using conditional statements gives you the opportunity to make decisions during program execution and gives you greater control over how the program works.
Practice
Here is an example of code that uses conditional statements in JavaScript:
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
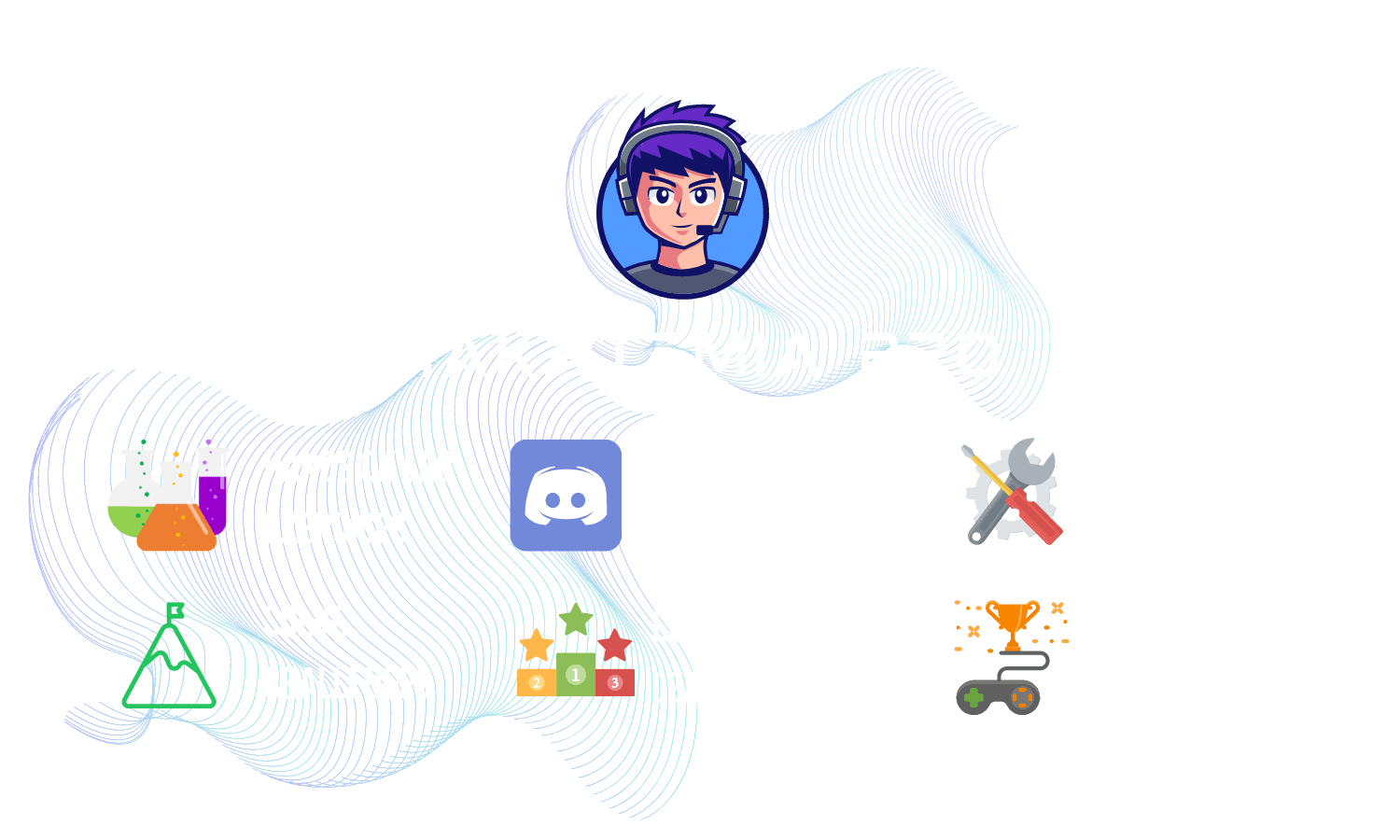
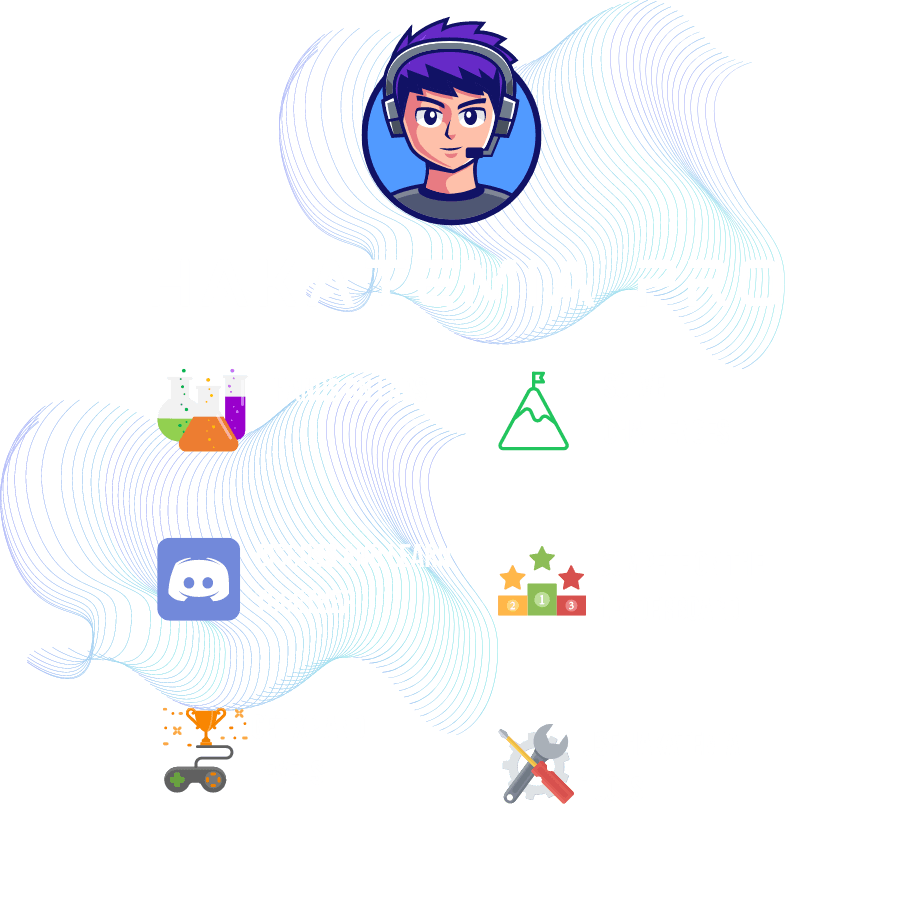
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.