Fetch and XHR are two ways to send network requests in JavaScript. Fetch is a newer API that has been available since 2015 and it provides a more modern and simpler way to send network requests. XHR (XMLHttpRequest) is an older way to send network requests and it has been in use since 2005.
Fetch
The Fetch uses the Promise interface, which enables easy management of asynchronous operations. The use of Fetch is simple, as it contains only one function that returns a Promise object. With this function, network requests can be sent and responses can be handled. For example:
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
XHR
XHR, on the other hand, uses callback functions, which are a bit more complicated to use. First, an XHR object is created, then desired parameters are set, and a network request is sent. When the response is received, it is processed with a callback function. For example:
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://jsonplaceholder.typicode.com/posts');
xhr.onload = function() {
if (xhr.status === 200) {
console.log(JSON.parse(xhr.responseText));
} else {
console.error('Error:', xhr.statusText);
}
};
xhr.onerror = function() {
console.error('An error occurred while sending the request.');
};
xhr.send();
Or if we want to send a POST request:
const xhr = new XMLHttpRequest();
const url = 'https://jsonplaceholder.typicode.com/posts';
const data = JSON.stringify({ title: 'Title', body: 'Content' });
xhr.open('POST', url);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onload = function() {
if (xhr.status === 201) {
console.log(xhr.responseText);
} else {
console.error('Error:', xhr.statusText);
}
};
xhr.onerror = function() {
console.error('An error occurred while sending the request.');
};
xhr.send(data);
In the example above, an XHR object is created, parameters such as request URL and data to be sent are set. Then the request is sent using the send() function, and the response is handled using the callback functions onload and onerror.
Which one to use?
If you have a modern browser that supports the Fetch API, it is highly recommended to use it due to its simplicity and efficiency. XHR, on the other hand, is useful in older browsers and for handling more complex network requests.
Practice
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
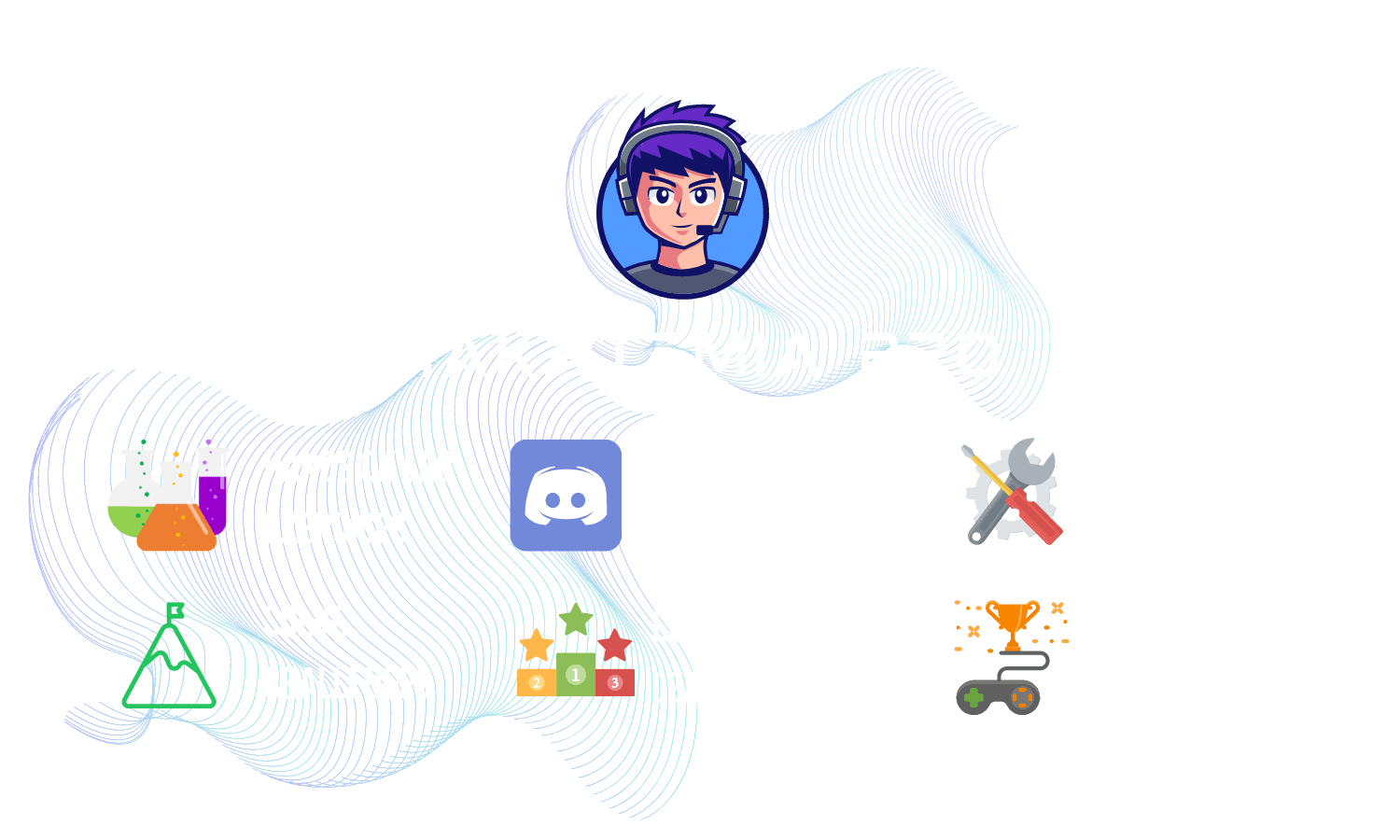
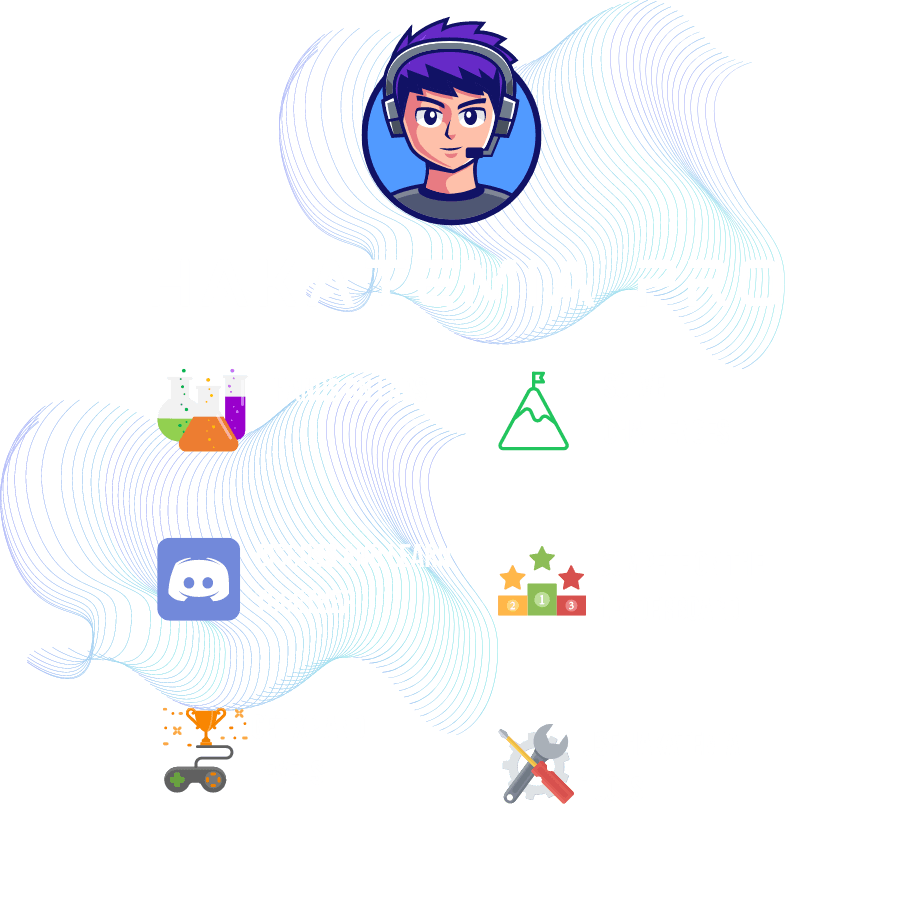
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.