Using functions is an important part of programming. Functions are chunks of code that can be called upon to perform specific actions when needed. This makes the code modular and easy to read. Here are some examples of using functions in JavaScript:
Example 1:
// Defining the function
function greet(name) {
console.log("Hello " + name + "!");
}
// Calling the function
greet("Matt"); // print "Hey Matt!"
In this example, a function named "tervehdi" is defined. The function takes one parameter, "nimi". The purpose of the function is to print a greeting message to the console, using the given name. When the function is called with the parameter "Matti", the console will print "Hello Matti!".
Example 2
// Defining the function
function calculateTotal(a, b) {
return a + b;
}
// Calling the function
var sum = countTotal(3, 5);
console.log(sum); // prints 8
In this example, a function laskeYhteen is defined, which takes two parameters a and b. The purpose of the function is to calculate the sum of these parameters and return it to the user. When the function is called with parameters 3 and 5, it returns 8, which is stored in the variable summa. This variable is then printed to the console, displaying "8".
The possibilities for using functions are limitless, and they can be used in many different ways to solve various problems. With them, the code can be easily readable and maintainable when tasks are divided into different functions that can be used when needed.
Practice
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
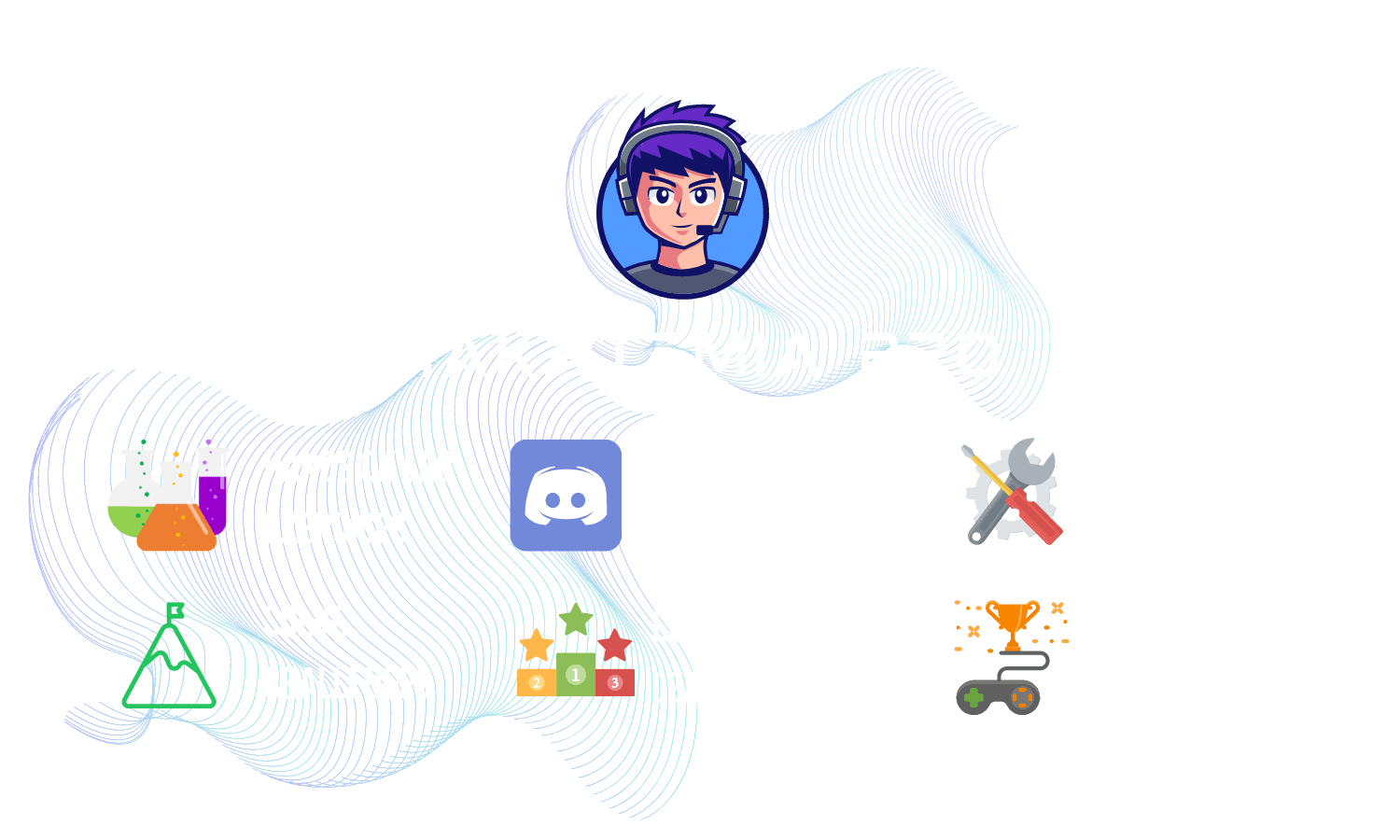
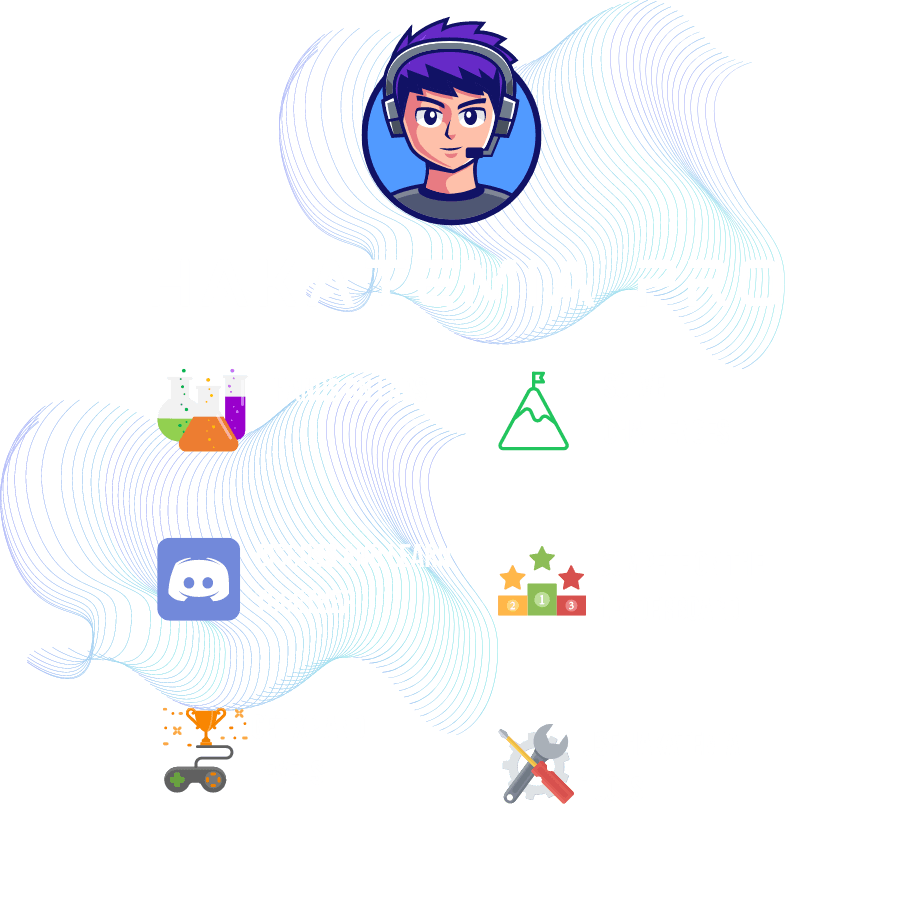
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.