Loops are programming structures that repeat a certain part of the code as many times as needed. This allows, for example, the repetition of the same operations multiple times, automation of code execution, and managing many other functions. JavaScript has several different types of loops, but the most common ones are for loops, while loops, and do-while loops.
for loops
For loops are usually used when you know in advance how many times you want to repeat a certain part of the code. The structure of for loops consists of initial conditions, condition statements, and increment statements. For example, you can use for loops to print numbers 1-10:
for (var i = 1; i <= 10; i++) {
console.log(i);
}
This defines the initial condition as "i = 1", the conditional statement as "i <= 10", and the increment statement as "i++". The loop repeats the code block 10 times, and with each iteration, the variable "i" increases by one.
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
while-loops
While loops repeat a certain part of the code as long as a certain condition is met. For example, you can use while loops to print numbers 1-10:
var i = 1;
while (i <= 10) {
console.log(i);
i++;
}
First, the variable "i" is set to 1. The while loop repeats the code as long as the variable "i" is less than or equal to 10. On each iteration, the variable "i" increases by one.
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
do-while loops
do-while loops work in the same way as while loops, but the code is executed at least once, even if the condition does not meet the requirements. For example, you can use do-while loops to print the numbers 1-10:
var i = 1;
do {
console.log(i);
i++;
} while (i <= 10);
In this code, the variable "i" is first set to the value 1. The code segment is executed once even if the condition is not met. The while loop checks the condition after the code has been executed at least once. The loop repeats the code segment as long as the variable "i" is less than or equal to 10. On each iteration, the variable "i" increases by one.
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
Summa summarum
Loops are an important part of JavaScript programming and are widely used in many applications and websites. It is important to understand the different types of loops and how they work so that you can use them effectively in your own projects.
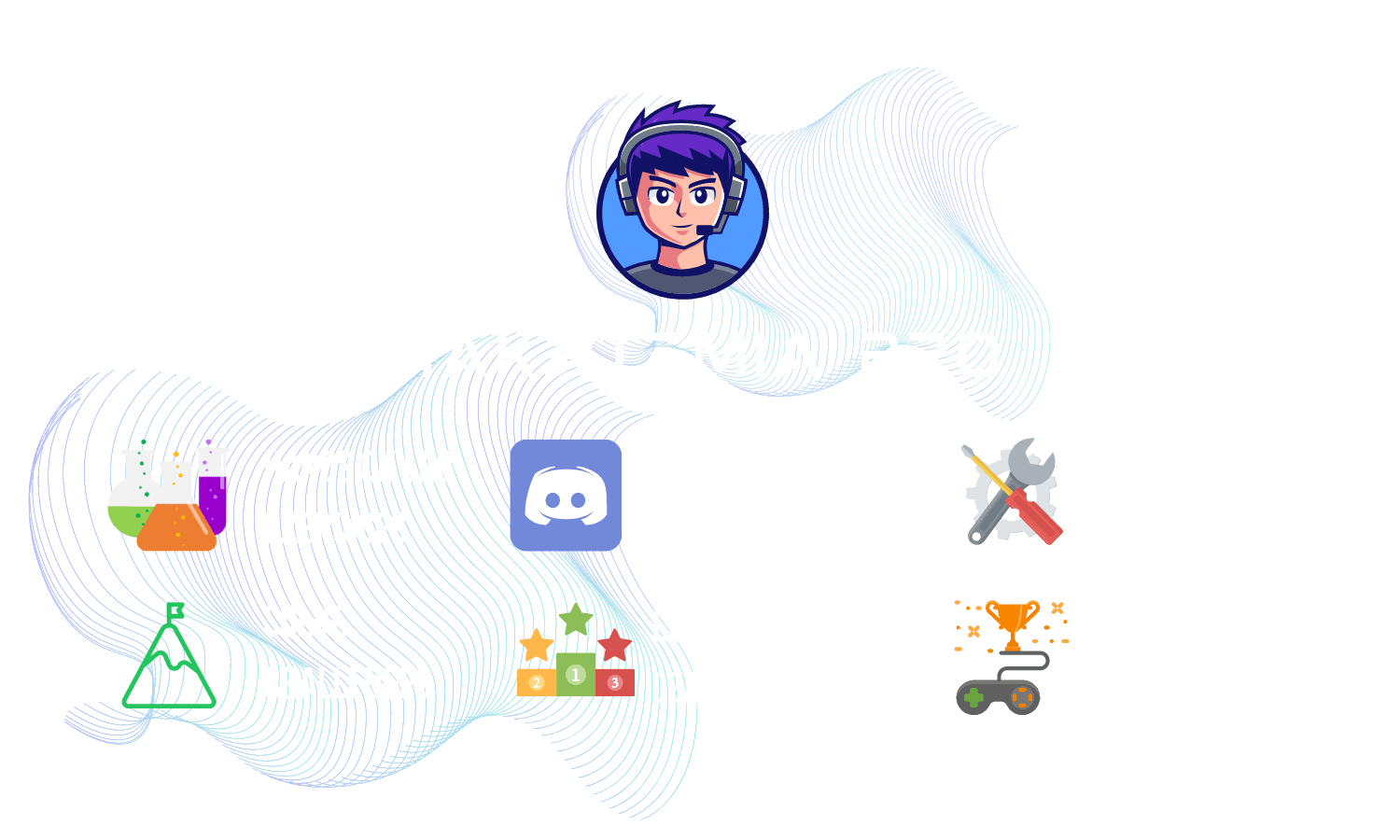
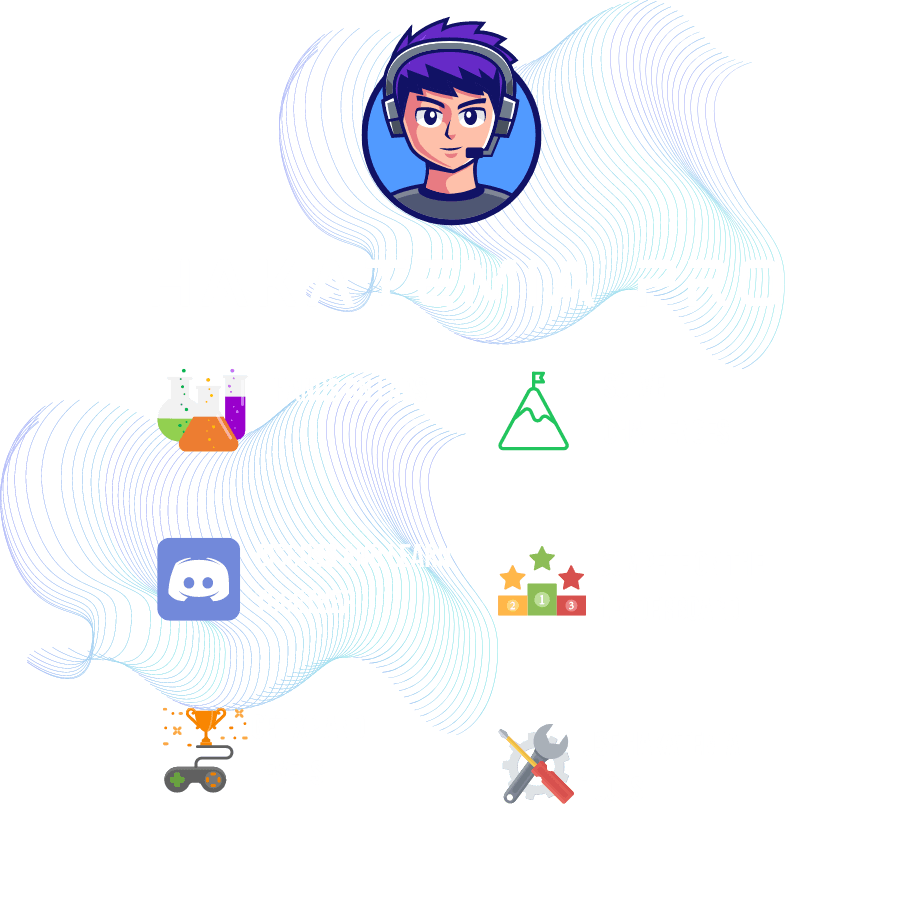
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.