Do you remember when earlier in the course we learned about HTML forms that send (usually POST-formatted) HTTP requests to the backend system? Let's now complement the equation by also building the backend side.
Simple Example
Let's start by creating a Flask application and defining a simple route that returns an HTML page with a form:
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
This route returns a template called 'index.html' which contains an HTML form. Let's create this template and add the form to it:
<!-- index.html --><!DOCTYPE html><html><head><title>Flask form</title></head><body><h1> Send data to Flask</h1><form method="POST" action="/submit"> <label for="nimi">Name:</label><input type="text" id="nimi" name="nimi"><br><br> <label for="sukunimi">Last name:</label><input type="text" id="sukunimi" name="sukunimi"><br><br> <label for="email">E-mail:</label><input type="email" id="email" name="email"><br><br><input type="submit" value="send"></form></body></html>
This form contains three fields: name, surname, and email. When the user submits the form, its data is sent with a POST request to the '/submit' route. Let's define this route in the Flask application and add code to handle the form data:
@app.route('/submit', methods=['POST'])
def submit():
name = request.form['name']
lastname = request.form['lastname']
email = request.form['email']
return f"Hello {name} {surname}, your email is {email}."
Practice
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
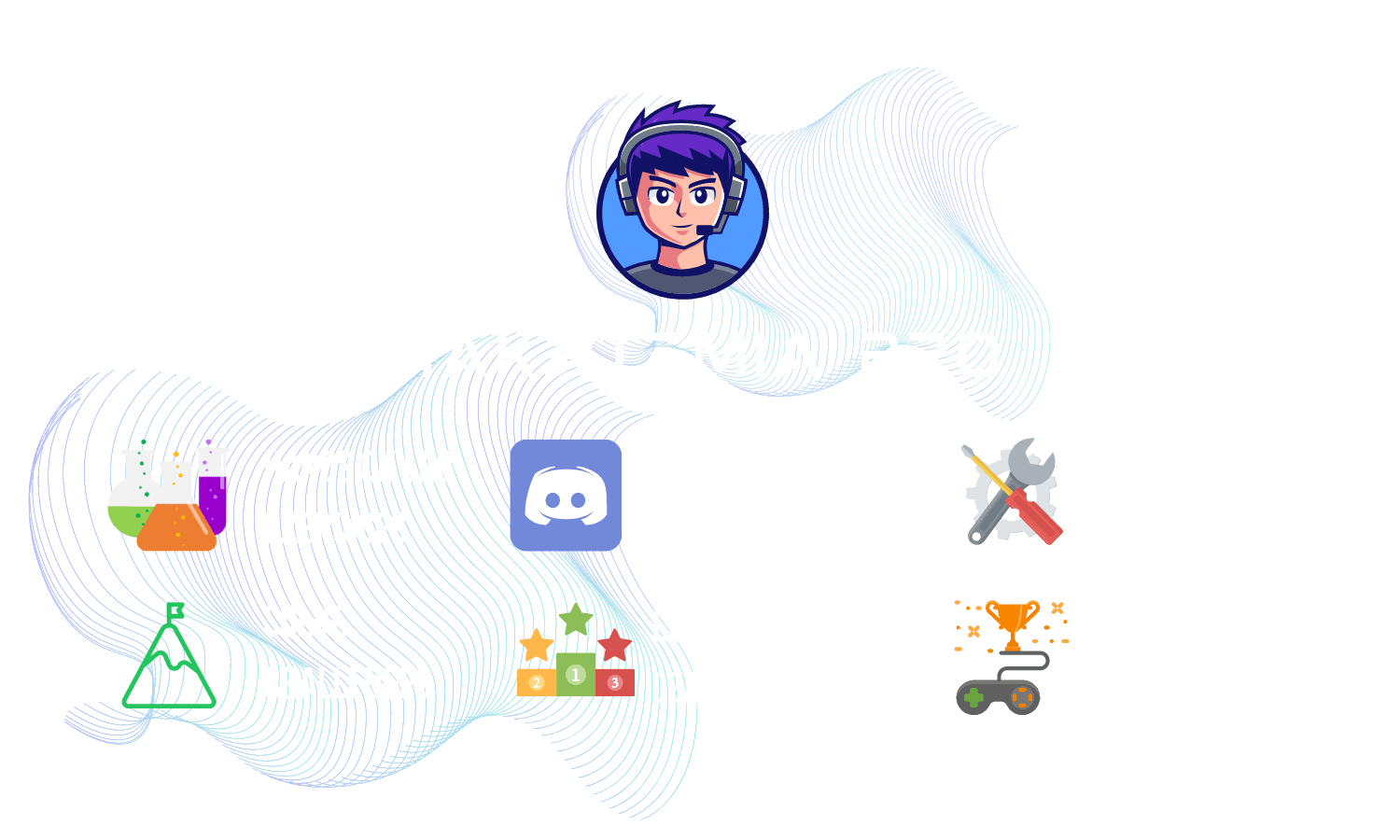
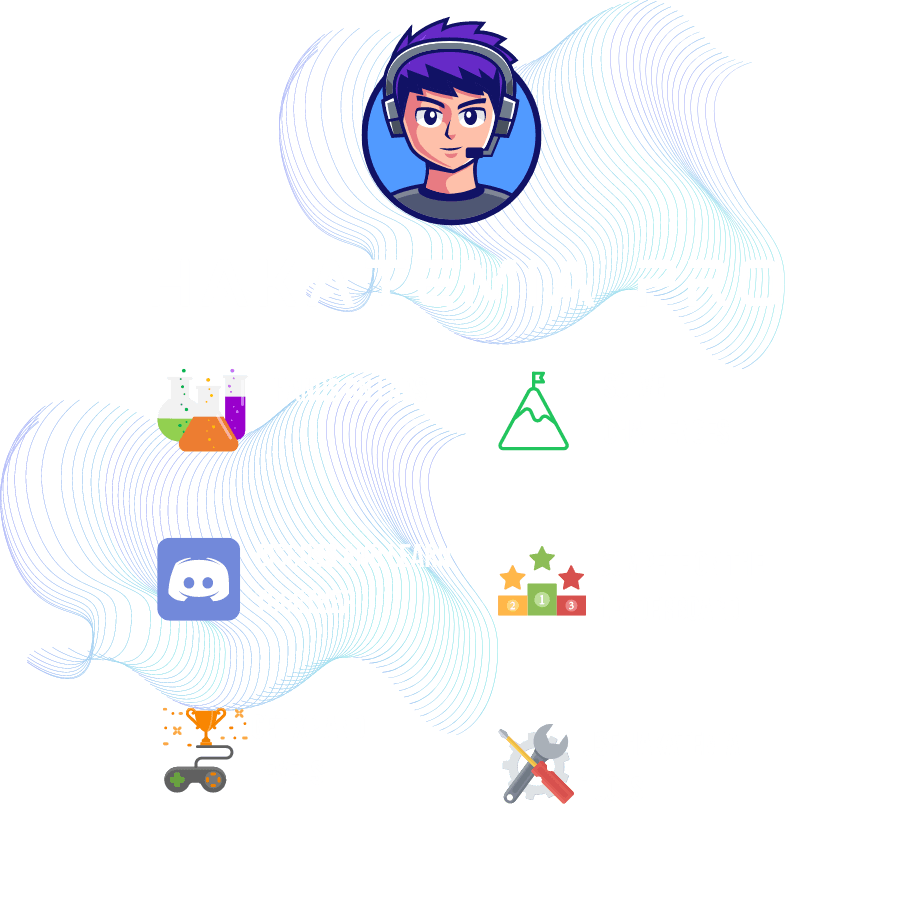
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.