Using JavaScript in an HTML document usually happens in two ways: by embedding the code within the HTML document or by referencing a separate JavaScript file.
Inline scripts
The first way to include JavaScript code in an HTML document is to use the <script> tag. This tag contains the JavaScript code that is executed when the HTML document is loaded. The tag is shown in the example in the head section, but it doesn't have to be exactly there. The head is loaded before the body, so if you want the script to be loaded only after the rest of the HTML has already been loaded, it is recommended to place it at the end of the body.
<!DOCTYPE html><html><head><title>Using JavaScript in an HTML document</title><script>
alert("Hei maailma!");
</script></head></html>
External scripts
Another way to use JavaScript in an HTML document is to refer to a separate JavaScript file. The JavaScript file is attached to the HTML document using the <script> tag, with the file location written in the src attribute. The script can be located at the application's own address (in which case it can be referred to with just the filename without a complete URL) or anywhere on the Internet.
HTML Document:
<!DOCTYPE html><html><head><title>Using JavaScript in an HTML document</title><script src="scripts.js"></script></head><body> <button onclick="tervehdi()">Say hello</button></body></html>
JavaScript file (scripts.js):
function greet() {
alert("Hello world!");
}
Note that functions in a separate JavaScript file are available in all parts of the HTML document as long as they are correctly linked to the HTML file.
DOM event handlers
HTML DOM event handlers are an important feature of JavaScript that allow us to define what happens when a user interacts with a webpage. DOM events can include mouse clicks, keyboard presses, or page loading.
Browsers also support DOM event handlers, which are defined in the attributes of HTML elements. This means that you can define an event handler directly in HTML instead of defining it in JavaScript code.
Here is an example of HTML code that defines an onclick event handler for a button element:
<!DOCTYPE html><html><head><title>DOM event handler</title></head><body> <button onclick="alert('Painoit nappia!')">Press me!</button></body></html>
In this example, an onclick event handler is assigned to a button element using an attribute. When the user clicks the button, the browser displays an alert window with the text "You pressed the button!".
Here is another example of HTML code that defines an onmouseenter event handler for a div element:
<!DOCTYPE html><html><head><title>DOM event handler</title></head><body><div onmouseenter="alert('Hiiri on nyt sisällä!')"> This is a div element</div></body></html>
Practice
Valitettavasti Replit-palvelu on muuttnut lennosta eikä enää anna suorittaa näitä koodeja suoraan selaimessa. Voit klikata alla olevaa "Open in Replit" linkkiä ja avata koodin Replit-palvelussa. Meillä on työn alla etsiä Replitille korvaaja.
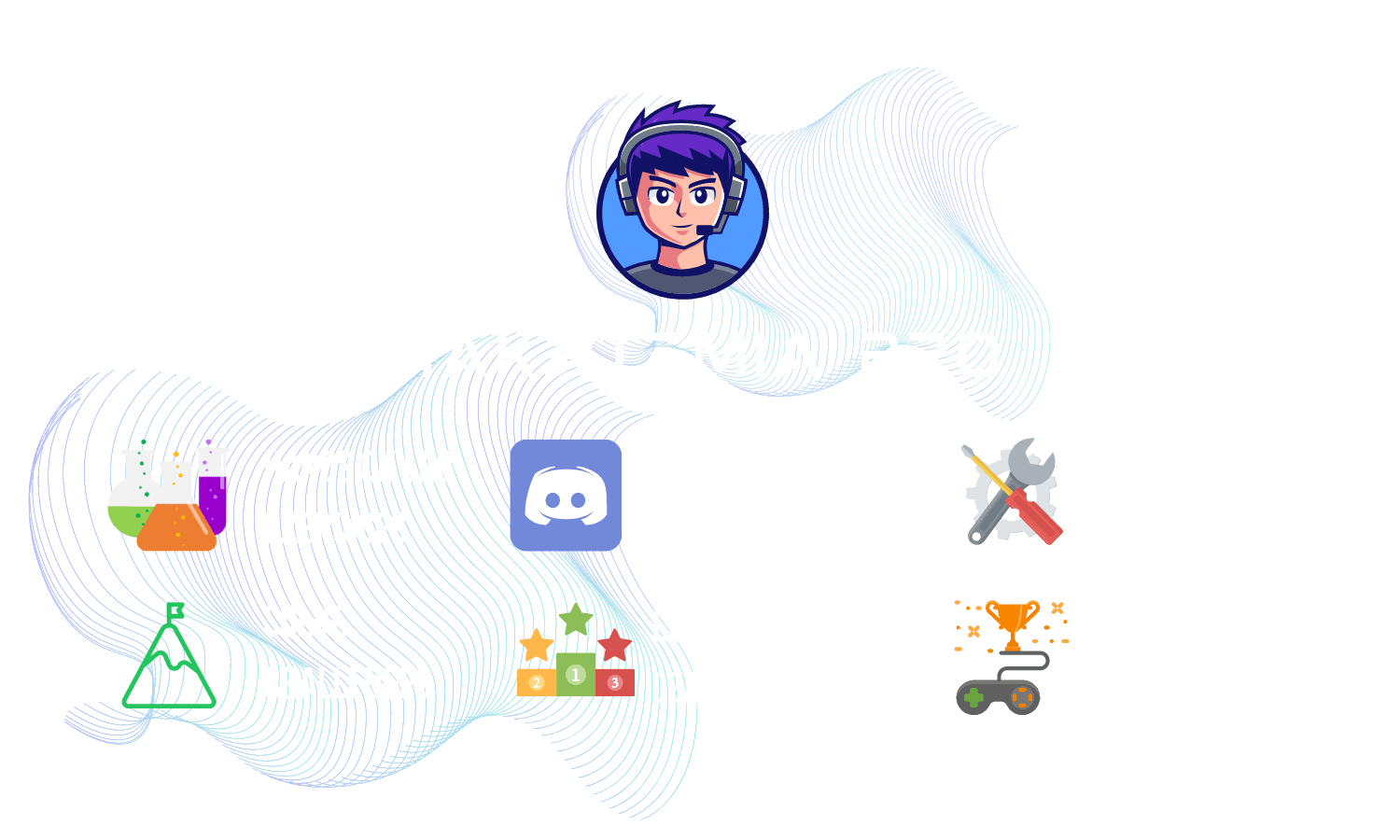
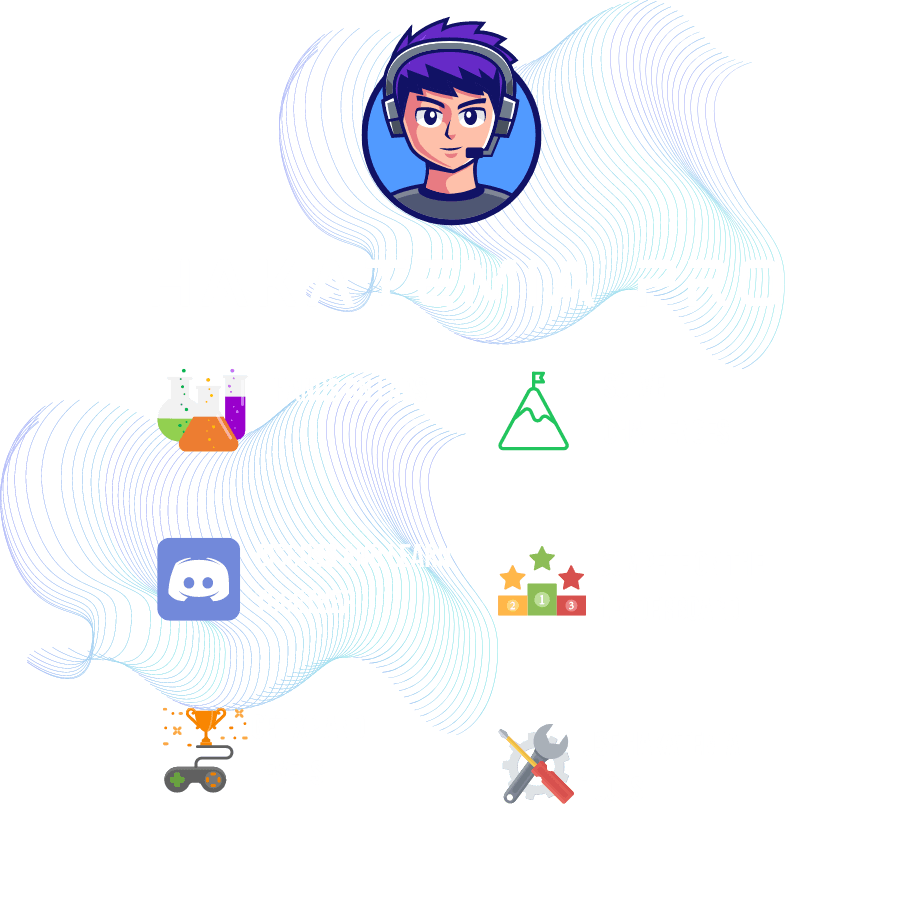
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.