Operating System Commands
In practice, almost all operating systems have some kind of command interpreter, a text-based interface. In Windows, it is called Command Prompt (cmd.exe) or PowerShell, in Linux and Mac it is bash, sh, zsh, etc. And these command interpreters can be used to start text-based programs and commands. For example, we can ping Google:
$ ping google.com
PING google.com (209.85.233.101) 56(84) bytes of data.
64 bytes from lr-in-f101.1e100.net (209.85.233.101): icmp_seq=1 ttl=114 time=82.4 ms
Operating System Commands in Programming
Sometimes applications utilize console tools in the background. We could have a web application, for example made with Python, whose purpose is to ping a specific IP address and display the ping result on the website. The code could look like this:
def do_GET(request):
ip = request.get_parameter("ip")
result = os.popen(f"ping -c1 {ip}").read()
return {"result": result}
Injection
The problem here is that adding user input to an operating system command is extremely dangerous. By adding certain special characters to the "IP address", an attacker may trick the application into executing completely different operating system commands than ping.
Command Substitution
Let's take command substitution, for example, which allows us to take the output of another command as part of a command. Substitution can be done with the syntax $(command) or `command`.
$ pwd
/ etc
$ echo "pwd is: $(pwd)"
pwd is: /tmp
$ echo "pwd is: `pwd`"
pwd is: /tmp
So by entering an IP address such as 1.2.3.4$(id) we could get the following response: "Ping 1.2.3.4$(id) result: ping: uid=0(root) gid=0(root) groups=0(root): Name or service not known." This is the output of the id command (in this case the user was root).
Imagination is the limit
There are countless ways to inject one's own commands in between or after if one is able to write within an operating system command. Some examples:
# Semicolon, starts a new command.
echo abc; id
# One pipe, takes the output of the previous command and redirects it to the new command
echo abc | id;
# Two ampersands, start a new command if the previous command succeeds.
echo abc && id;
Exercise
Labrassa is a web diagnostic tool that checks if a website is up. The tool uses the curl command in the background. From the top of the page, you can see which commands are executed on the server.
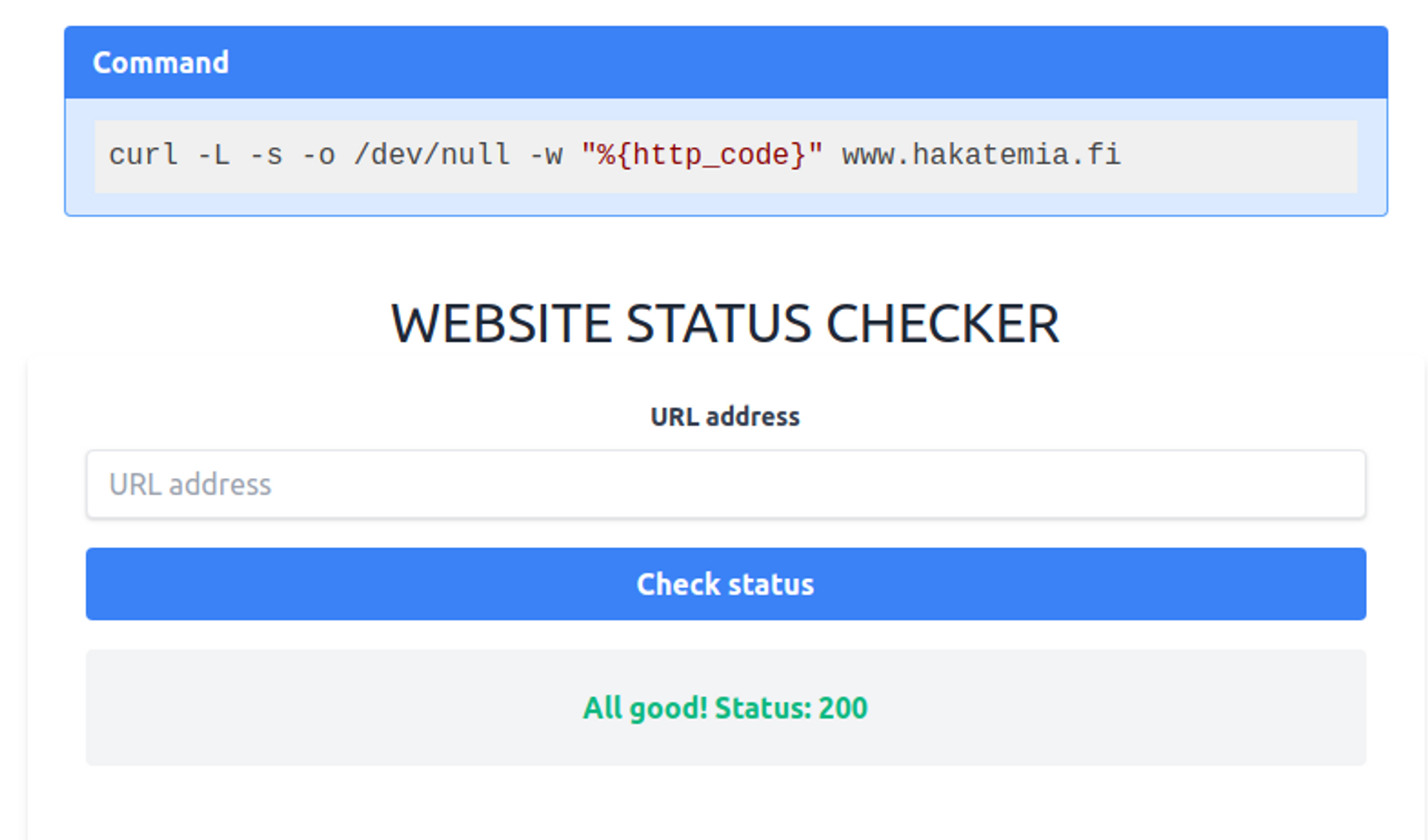
Try to read the file /flag.txt.
It is generally advisable to avoid executing operating system commands and strive to achieve the same result with code. If this is not a preferred option, it is important that:
1. Operating system commands are never constructed by concatenating text, like:
os.system("ping " + ip)
The commands should be built using a software library that securely ensures that the input does not spread beyond the specified parameter:
subprocess.Popen(['ping', ip])
2. The parameter that comes from outside the program (such as user input, database, API, etc.) must be validated as accurately as possible.
3. The parameter that the user of the application is allowed to influence, for example, must be properly understood. Let's take curl as an example. Is it enough to use a secure library to form the curl call and let the user only decide the URL address? Should we also validate that the URL is in the correct format?
Not enough! With Curl, you can read files from the server's disk.
$ curl file:///etc/passwd
root:x:0:0:root:/root:/bin/bash
daemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologin
What if you ensure that the URL addresses start with http:// or https://? Not enough! With cURL, one could call local or intranet HTTP interfaces on the server that were not meant to be open to the Internet.
The point is, that operating system commands often have capabilities that one wouldn't have thought of. If they have to be used, it is important to exercise caution.
Online diagnostics
In this lab, you get to practice command injection attack against a vulnerable network diagnostic service.
Objective
Acquire root permissions on the server and read the file /flag.txt (for example, with the cat command).
Exercises
Flag
Find the flag from the lab environment and enter it below.
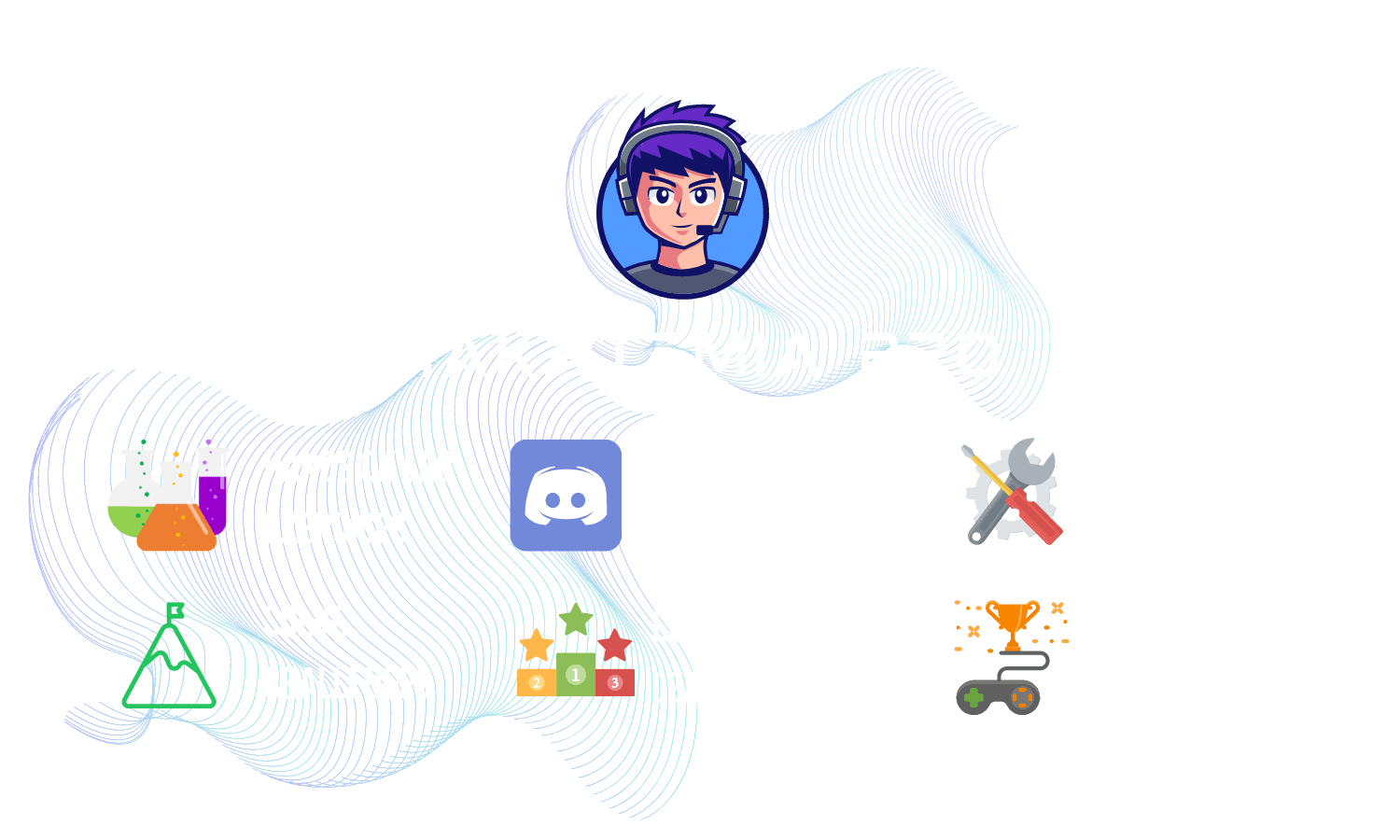
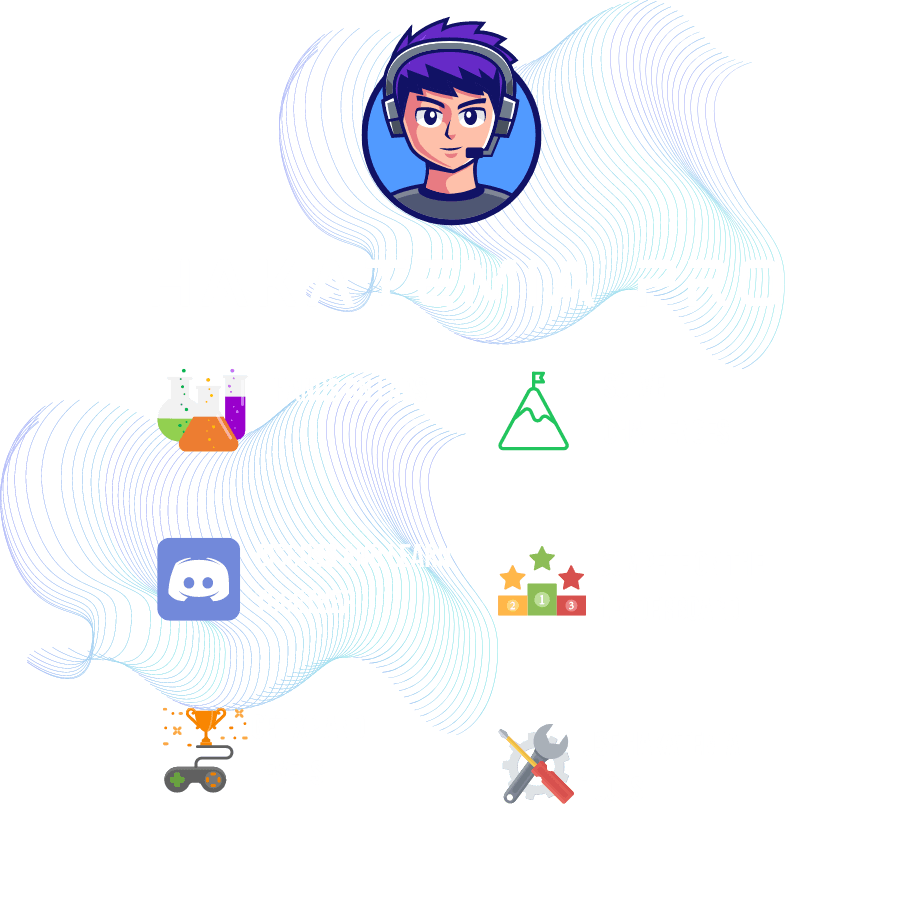
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.