What is an Initialization Vector (IV)?
Initialization Vector (IV) is a random bit string used in cryptographic operations, such as encryption and decryption, to enhance security and prevent certain attacks. It acts as an additional "salt" that is mixed with the message itself to produce a unique ciphertext each time.
Do not reuse IV
It is extremely important that the same IV is never used twice. Each message to be encrypted must use a unique and unpredictable IV. If the IV is reused, attackers can exploit this vulnerability to decrypt messages or manipulate their content.
Example
Below is the Python example code that dangerously uses an Initialization Vector (IV) in AES-CBC encryption mode. In this example, the Initialization Vector (IV) is set to a fixed value, which is a bad practice:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
import os
# Suppose we have data to encrypt
data_to_encrypt = b"Secret message that needs encryption"
# Using fixed IV (bad practice!)
iv = b"1234567890123456"
# Generate a random encryption key
key = get_random_bytes(16) # AES requires the key to be either 16, 24 or 32 bytes long
# Create an AES-CBC cipher object
cipher = AES.new(key, AES.MODE_CBC, iv)
# Encryption
encrypted_data = cipher.encrypt(data_to_encrypt.ljust(16, b"\0")) # Data must be filled in the correct block size
Vulnerability in the use of fixed IV
The use of a fixed Initialization Vector (IV), especially using a publicly known value, is a security risk in cryptography. It causes the following vulnerabilities:
Predictability: When the IV is fixed or predictable, an attacker can predict the encryption of the first block before the encryption process begins. This significantly reduces the security of encryption, as the attacker can prepare for possible attacks knowing how the encryption starts.
Same messages produce the same encryption: If the same IV and encryption are used repeatedly, the same original messages produce identical encrypted messages. This can reveal information about the content of messages and enable pattern-based attacks, such as chosen-plaintext attacks.
Revealed beginning of the message: In particular, in CBC mode, the encryption of the first block depends only on the IV and the original message. If the attacker knows or can guess part of the original message, using a fixed IV can make it easier to decrypt the rest of the message.
Use IV correctly
The correct practice is to use a cryptographically secure randomly generated IV for each encryption operation. IV generally does not need to be secret, but it should be unpredictable and unique for each encryption round. This significantly improves security, preventing the vulnerabilities mentioned above.
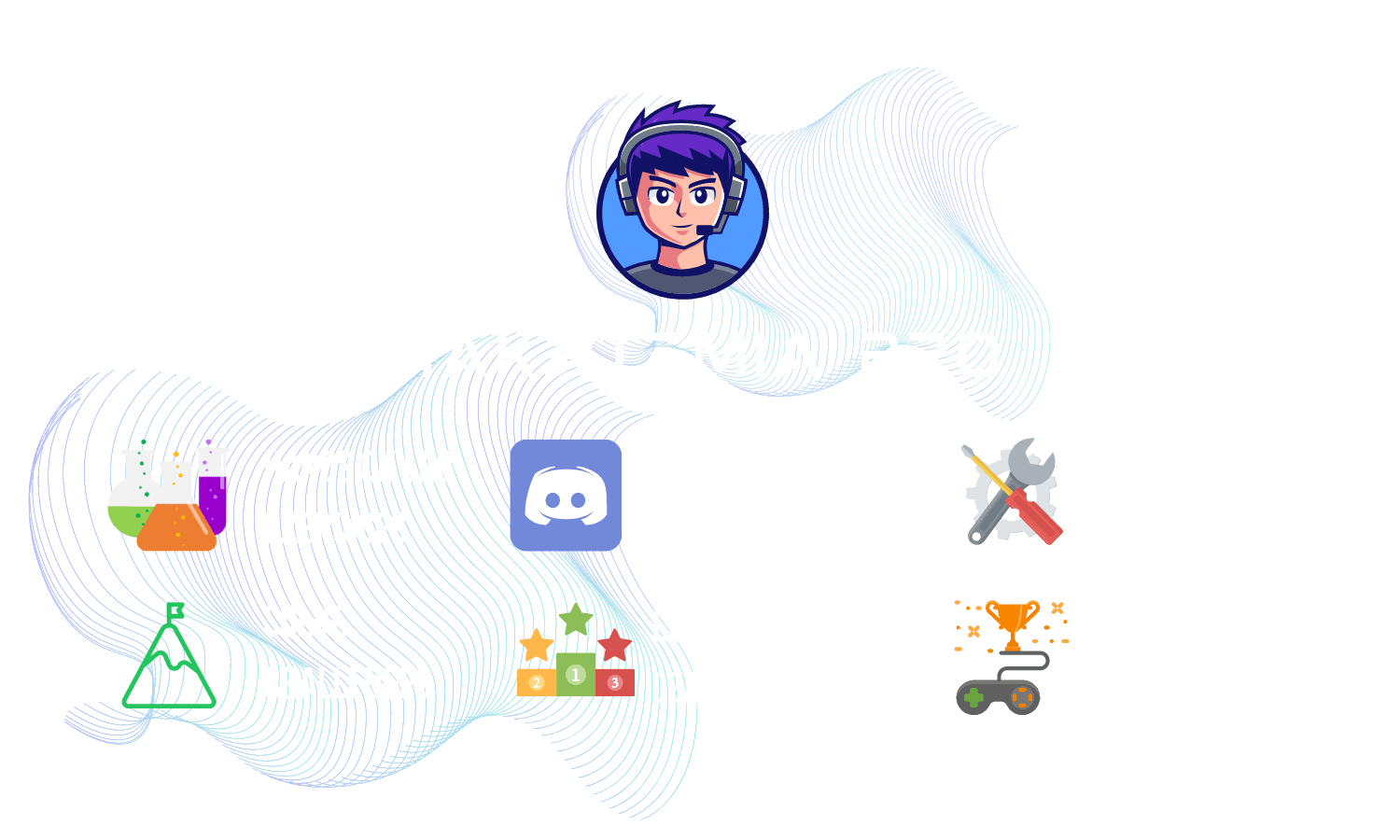
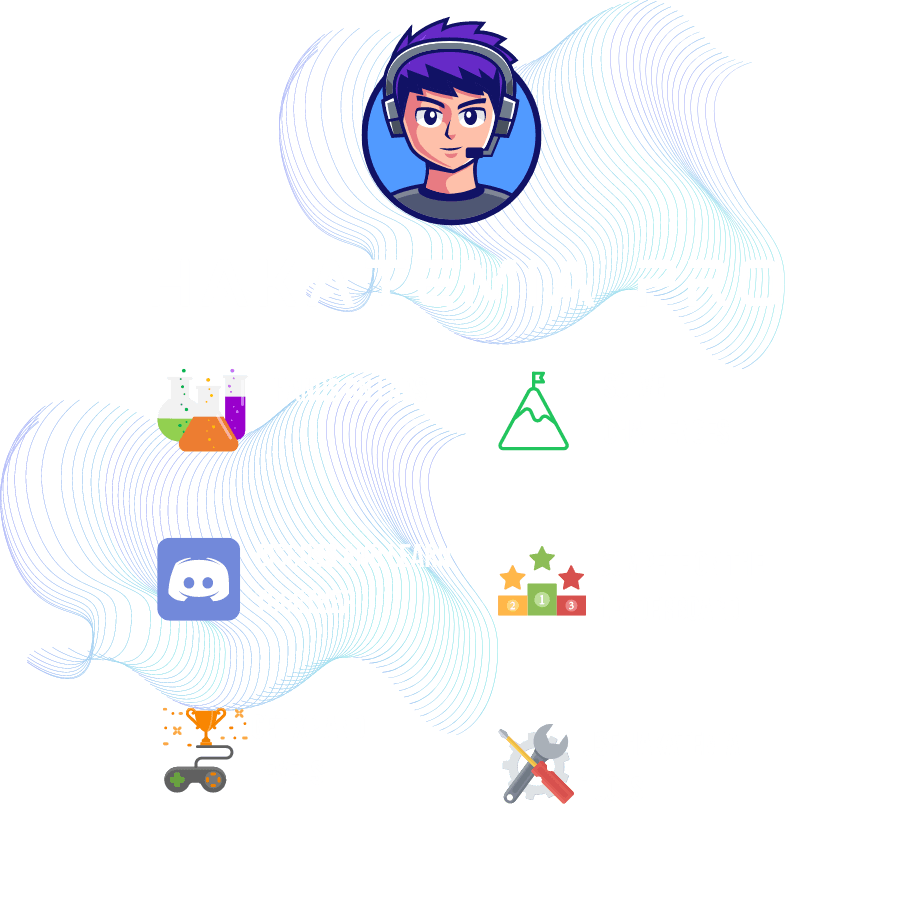
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.