In this module, a simple webpage is built with the Flask library. Understanding the content of this module will greatly help in solving the final assignment of the course. This module also mainly focuses on the server-side, and not much development is done on the browser side or the "frontend."
Routes
Everything starts with routes. Routes refer to the mappings of URL addresses or paths used in the browser to functions written in the code. Let's take an example.
https://www.example.com/route_1/
When the browser visits the example address, the website must have a way to distinguish and determine what happens when that address is loaded. This could look like the following in the code:
route("/route_1")
def route_1_function():
return(route_1.html)
In the pseudocode above, we have created a route for the website that handles the functionality of the URL address in a way that it returns the route_1.html file to the browser when the browser opens this address.
This is perhaps the most important concept to understand in the whole topic area. Websites can be built in different ways using various frameworks and programming languages, but they all have the same need. In every solution, we must be able to map the addresses visible and loaded in the browser to the code running on the server-side of the website.
Flask Application
Keeping the above concept in mind, let's now write our own Flask web application in Python. You can do this either on your own device right now or simply follow along.
from flask import Flask
app = Flask(__name__)
@app.route('/')
def homepage():
return '<h1>Welcome!</h1>'
@app.route('/help')
def help_page():
return '<h1>Help is here!</h1>'
if __name__ == '__main__':
app.run()
The above example is now a functioning Flask application with two routes defined. These route mappings occur within @app.route, under which the specific functionality to be executed when this route is loaded is programmed.
What is not a functioning route in the example above?
In both routes, nothing more happens than the application returning to the browser or the "frontend" HTML code with a simple text snippet. Note the return.
These can typically be much more complex, as at this point in the code, actions such as
- user authentication (does the user have the right to access this page)
- fetching information from other services or a database, which is then included in that returning HTML code
- Making changes to the stored information, etc., etc.
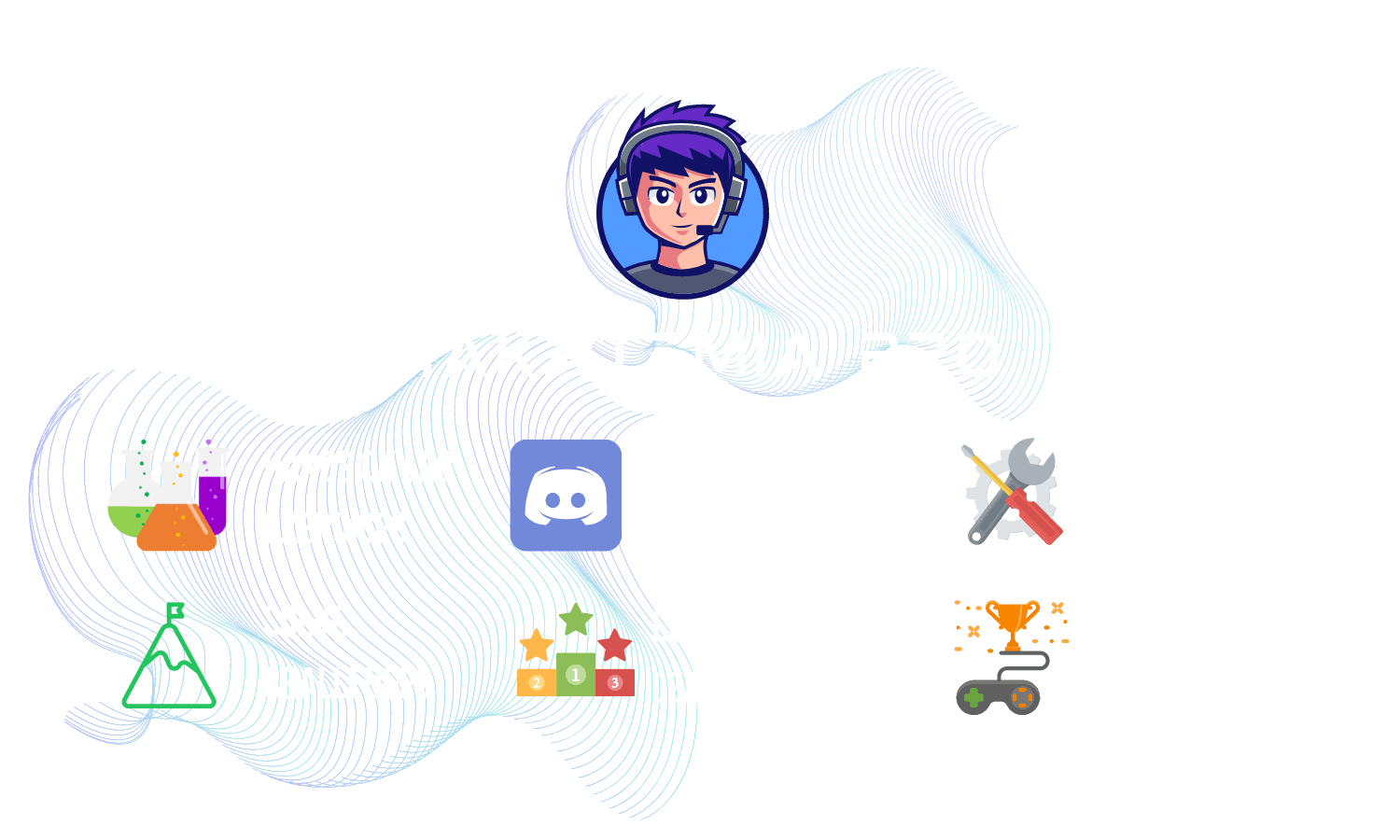
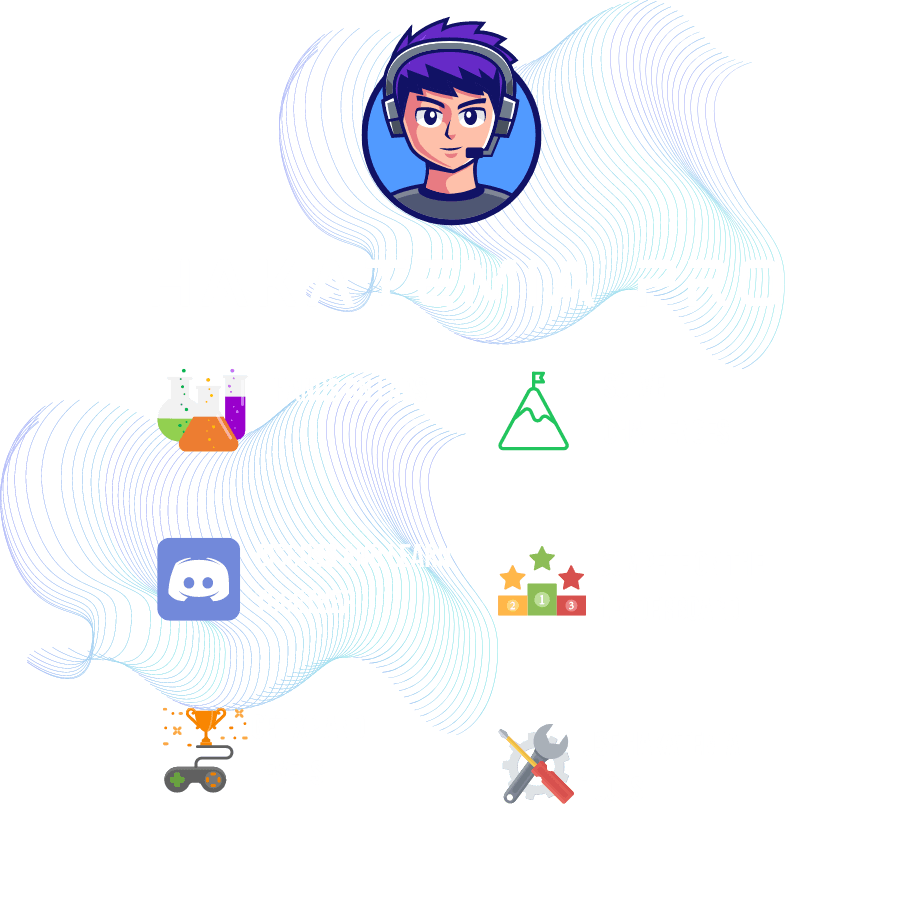
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.