The for loop is another fundamental tool in Python programming that allows for the repetition of a specific code block multiple times. The for loop can be used to iterate through all the elements of a list, dictionary, or any other iterable object, one element at a time, and execute the same code block for each element.
The general syntax of a for loop is as follows:
for variable in iterable_object:
# executable code
"muuttuja" is a variable that gets the value of the next element of the iterated object on each loop iteration. "iteroitava_objekti" is any iterable object, such as a list, dictionary, or some other data structure, that contains multiple elements.
For instance, if you want to print each item of the list one by one, you can use the following for loop:
list = [1, 2, 3, 4, 5]
for number in list:
print(number)
This would print the following:
1
2
3
4
5
The for loop is very useful in many situations when you need to repeat certain code blocks multiple times. You can use the for loop, for example, in processing file lines, processing database records, and many other situations.
Exercise
Define a list named x that contains at least 3 numbers. Define an empty list named y. Write a for loop that iterates through all the numbers in list x and adds them to list y doubled.
Example of how the exercise should work.
x = [1, 4, 10]
y = []
# make a for loop here
print(y)
[2, 8, 20]
Please be careful with variable names, as automatic checking may not succeed. Printing is optional, the most important thing is the correct construction of variable y.
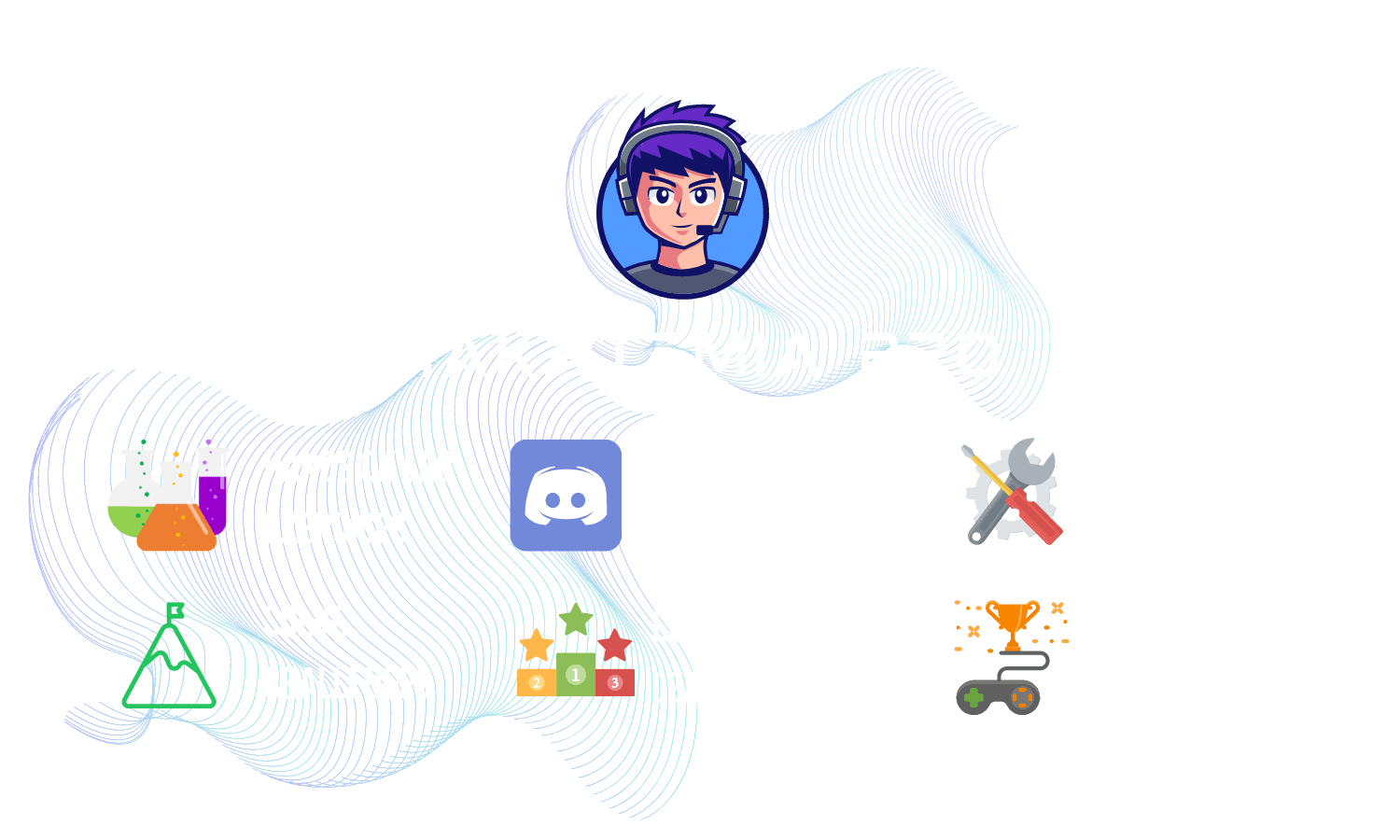
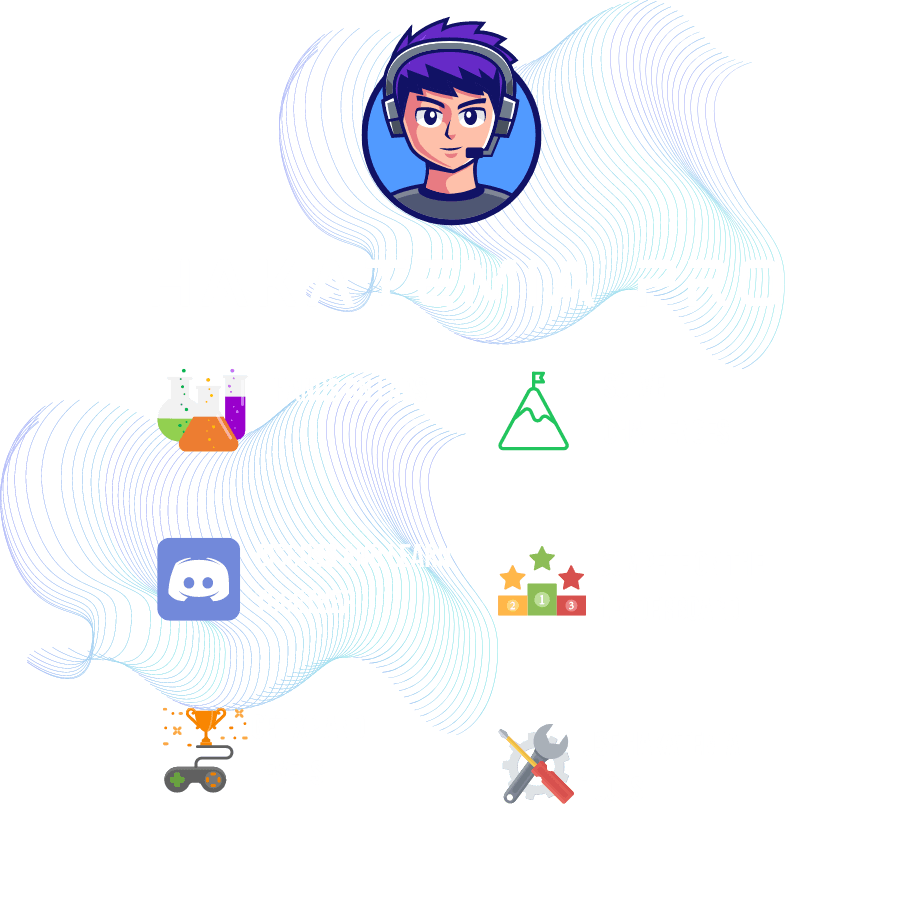
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.