Loops
Basic programming includes loops. Loops are repetitive structures that perform certain functions multiple times. The while loop is one of the most common loops and it repeats certain functions until a certain condition is met.
while loop in Python
while loop repeats actions as long as a certain condition is true. The program first checks if the condition is true, and then performs the loop. After the loop, the program checks the condition again and performs the loop if the condition is still true. The loop continues until the condition is no longer true.
For example, if you want to repeat an action five times, you can use a while loop as follows:
i = 0
while i < 5:
print("This is repeated five times.")
i += 1
This program prints the text "This is repeated five times." five times, because the while loop repeats the action until i is greater than or equal to 5.
The program's operation is explained as follows:
- The i variable is initially set to 0.
- The program first checks if i < 5, which is true because i is 0.
- The program prints the text "This repeats five times."
- The i-variable is incremented by one.
- The program checks again if i < 5, which is true because i is now 1.
- The program prints the text again and increases the value of i.
- This will repeat until i is greater than or equal to 5.
Applications for the while-loop
The while loop can be used in many different situations. For example:
- Data input: the while loop can be used to repeat data input until the user provides the correct input.
- Enumeration: the while loop can be used for enumeration or generating numbers when a specific condition is met.
- Reading files: a while loop can be used for reading files until the file has been completely read.
Let's look at the following example that asks the user for numbers until the user enters a negative number. The program also calculates the sum of the entered numbers.
sum = 0
while True:
number = int(input("Enter number: "))
if number < 0:
break
sum += number
print("The sum of the numbers is", sum)
In this program, the while loop continues indefinitely (because the condition is True) until the user enters a negative number. Inside the loop, the user is asked for a number and the sum is calculated. When the user enters a negative number, the program breaks the loop with the break statement and prints the final sum.
Exercise
Write a program that asks the user for numbers until the user enters two consecutive same numbers. After that, the program will output the sum of the entered numbers, including the last number.
For example:
Enter a number: 1
Enter the number: 3
Enter the number: 5
Enter the number: 3
Enter the number: 2
Enter the number: 2
The sum of the numbers is 16
Conclusion
The while loop is a useful tool in Python programming. It allows you to repeat certain code blocks as long as certain conditions are met. Combined with other programming elements such as conditional statements, the while loop can help programmers implement complex programs.
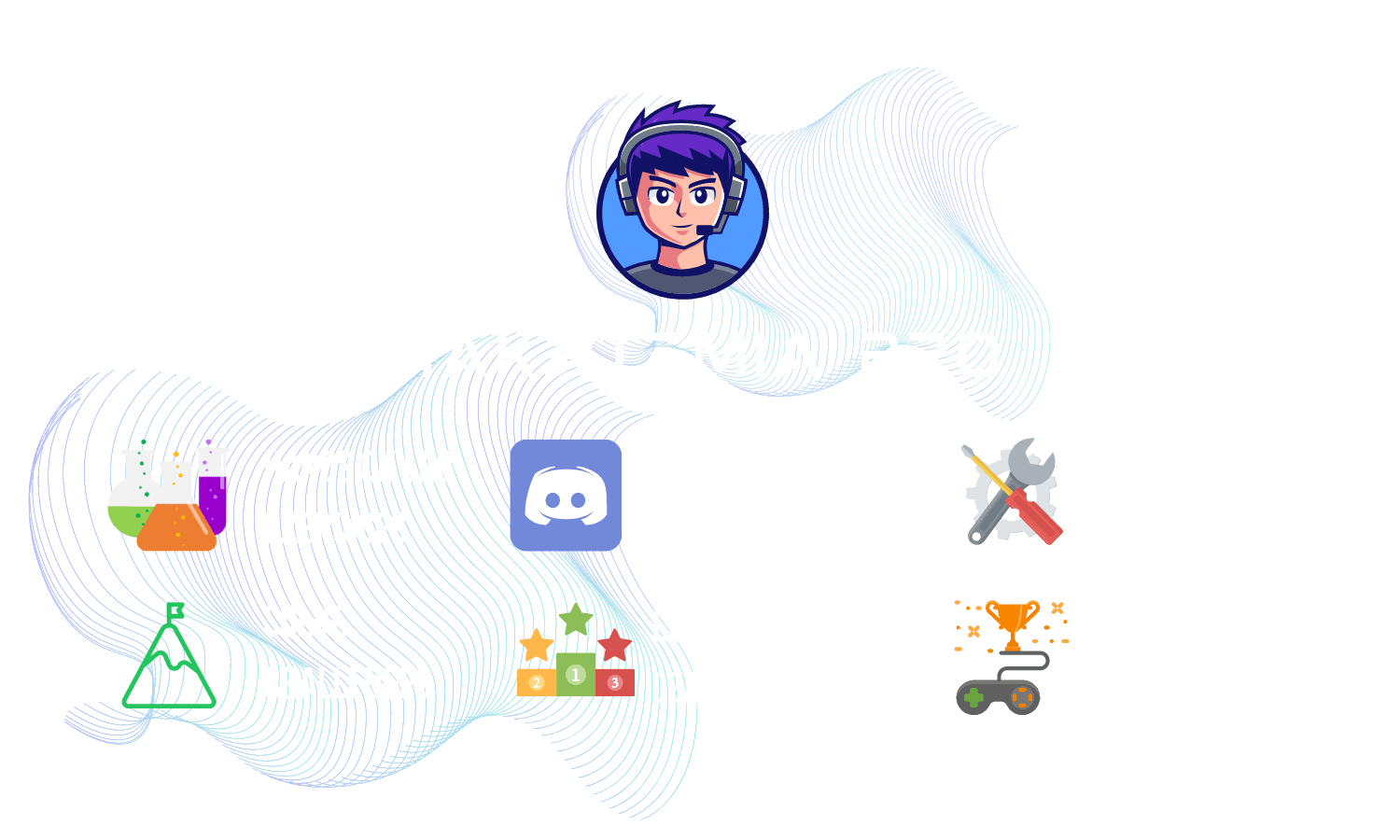
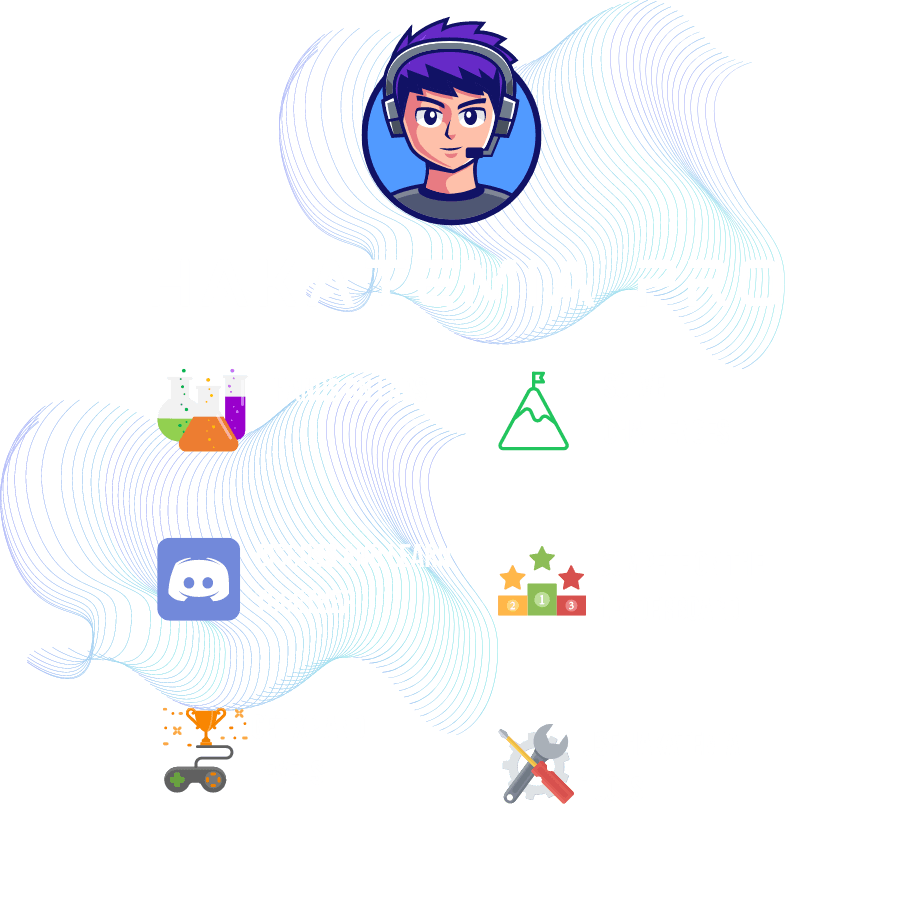
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.