Cobra Mathematics!
In Python code, you can easily perform calculations. Let's print the sum of the arithmetic operation 3+3 as an example.
print(3+3)
6
Or, let's create a variable sum, whose value is the sum of the calculation 3+3.
sum = 3+3
print(sum)
6
Variable computation
Let's take an example of variables from the previous module:
player1_hp = 100
player2_hp = 100
We can easily create a variable whose value is the sum of both players' HP.
total_hp = player1_hp + player2_hp
Adding or Subtracting the Value of a Variable
What if player 1 took 10 damage and we wanted to decrease the player's HP by ten? This can be done by giving the player1_hp variable a completely new value, but using the original value as a variable in the new value, subtracting 10 from it.
player1_hp = player1_hp - 10
In Python, there is a small shortcut for this (it does the same thing as above):
player1_hp -= 10
Boosting works the same way, let's say that the other player casts a healing spell that heals 10 damage.
player1_hp = player1_hp + 10
Or alternatively shortcut:
player1_hp += 10
Multiplication and division operations
Multiplication operations are performed using asterisks (*) and division operations using forward slashes.
print(6 * 7)
42
print(12 of 4)
3
Exercise
Just to do the task! You can use variables x and y with any numbers you want.
Exercises
Task 1
Make the variables x, y and sum. Define the value of the variable sum as the sum of the variables x and y (i.e. the result of the addition).
Task 2
In addition, make the variable income. Define the product of the variable as the product of the variables x and y (i.e. the result of multiplication).
Task 3
In addition, variable separation. Define the variable difference as the difference between the variables x and y (ie the result of the subtraction).
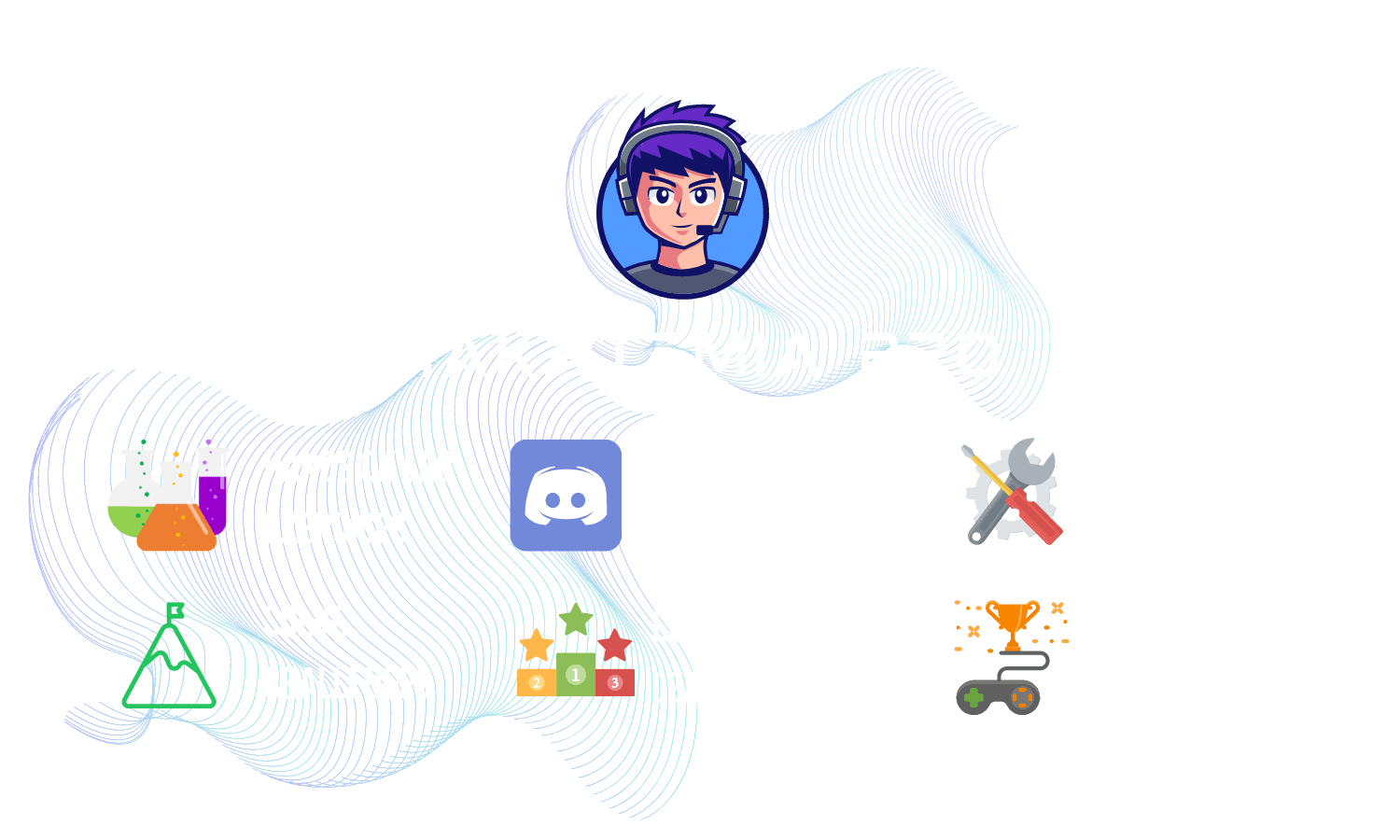
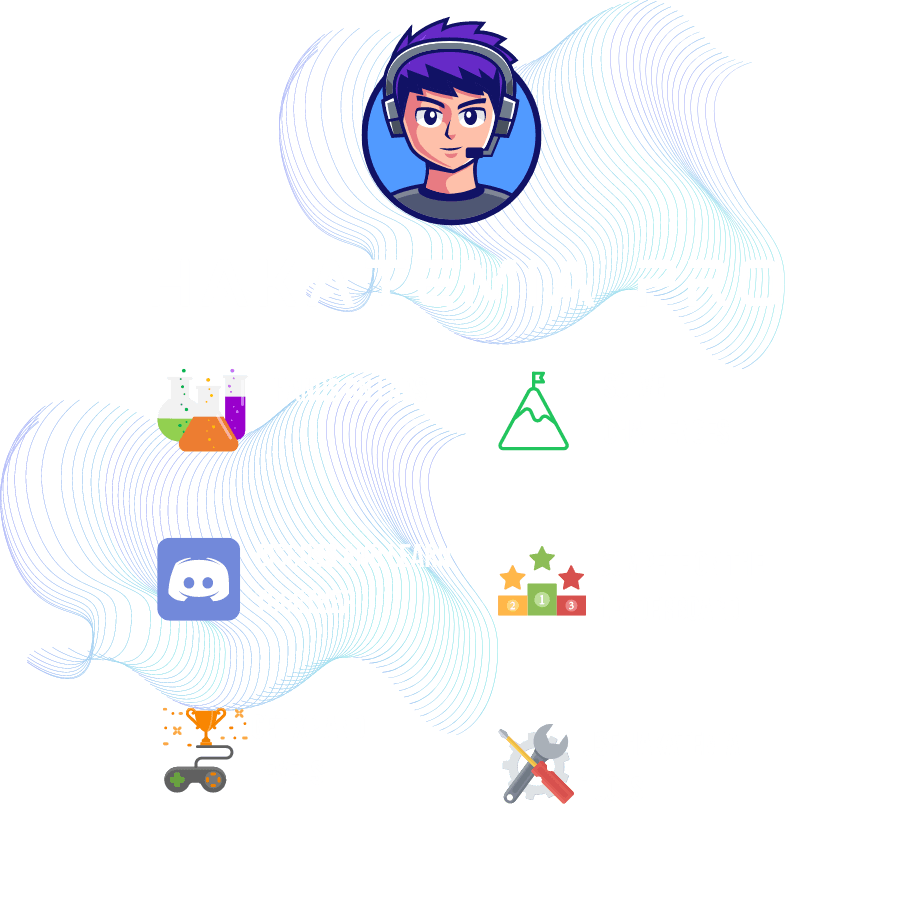
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.