What are conditionals?
Let's say we have a game that ends when a player's HP drops to zero or below. Then we need to check the value of the variable player1_hp and if it is zero or below, we go to the "game over" section in the code. For example, like this:
player1_hp = 50
if player1_hp <= 0:
print("Game over")
The program works as follows:
- Define a variable player1_hp and assign it the value 50.
- Checking if the value of player1_hp is less than or equal to (<=) 0.
- If yes, print "Game over".
So IF SOMETHING then DO SOMETHING. Here are two important things about Python: Indentation and Blocks.
Indentation and Blocks
In Python code, there are blocks, "chunks", that are executed only under certain conditions. In the example above, the block
print("Game over")
It is executed only if the preceding conditional statement is true. The block is identified by the fact that:
- It is preceded by a colon at the end of the previous line.
- It is indented further to the right from the previous line.
In Python, unlike many other programming languages, indentation actually matters.
if, else
In conditional statements, it is also possible to define another block, that is, what happens if the condition is false. This is done using else:
player1_hp = 50
if player1_hp <= 0:
print("Game over")
else:
print("Player is still alive")
elif
You can actually put as many blocks as you want in conditionals, using elif statements.
player1_hp = 50
if player1_hp <= 0:
print("Game over")
elif player1_hp < 10:
print("The player already has one foot in the grave.")
else:
print("The player is still healthy.")
The above program works as follows:
- If player1_hp <= 0, output "Game over".
- If not, and if player1_hp < 10, print "The player is already one foot in the grave".
- If not, then reduce until "The player is still in good shape."
Note that these blocks will always lead to only one option.
Comparison of Numbers
- > Greater than.
- < Less than.
- >= Greater than or equal to.
- <= Less than or equal to.
- == Equals.
Exercise
Example of how the program should work:
Enter the number: 5
equally
Enter the number: 3
under
Enter the number: 9
over
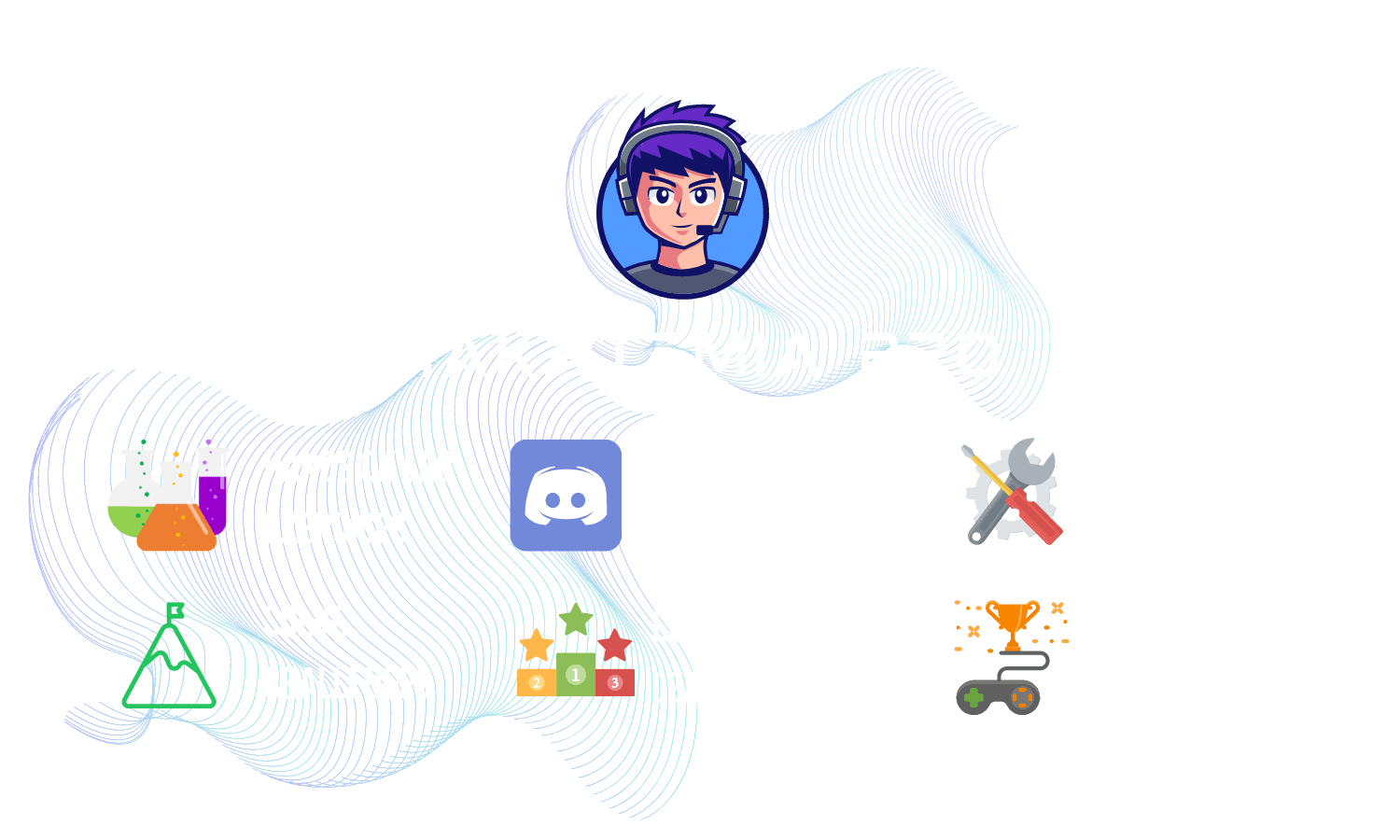
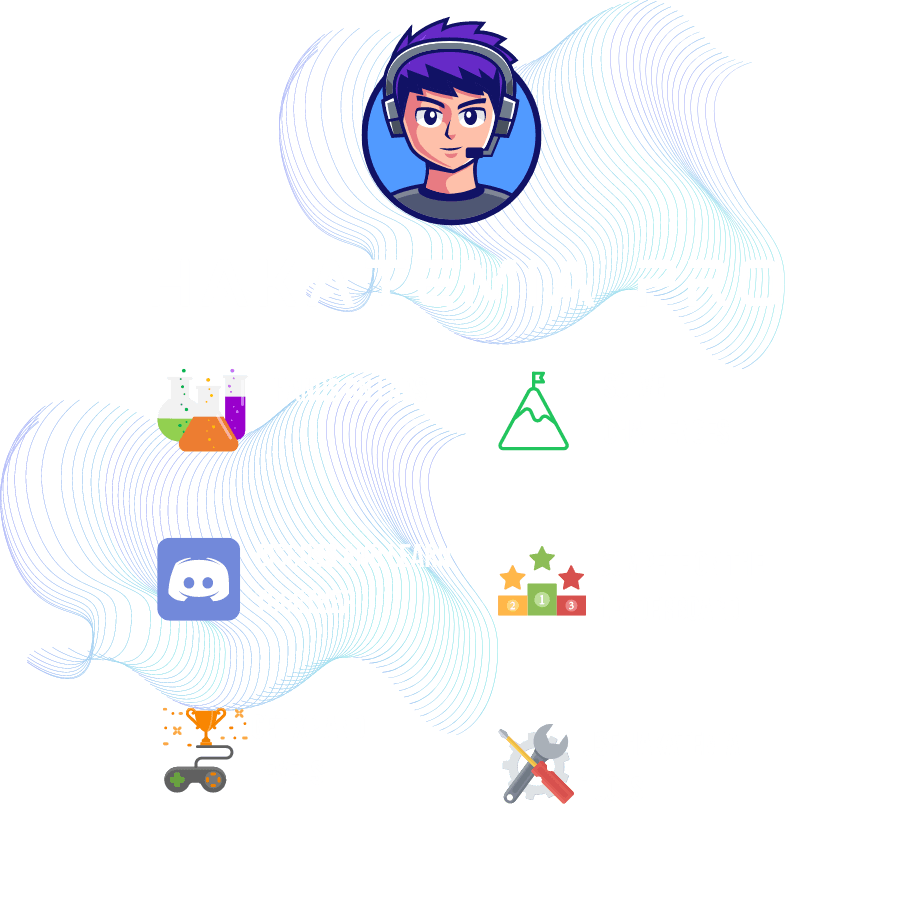
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.