What are data types?
Data, such as variables, is not always of the same type. For example, if you concatenate two variables of type text, the result is that the texts are joined together.
a = "3"
b = "5"
print(a + b)
35
On the other hand, if the variables are numbers, the result is the sum of the numbers.
a = 3
b = 5
print(a + b)
8
And if you try to perform an operation with two types that are not compatible, you will get an error.
a = 3
b = "5"
print(a + b)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'str'
This is Python's way of displaying error messages, which you should get used to. You can see from the message what the error was and on which line it occurred. int means number (Integer) and str is the data type for text (String). The error in this case is incompatible operands (left and right side) for the plus operator.
What types of data are there?
There are quite a few different data types and you can also define more yourself. Here are the built-in data types in Python and roughly their purpose. You don't need to remember these yet, but take a look.
- Text: str
- Numbers: int, float, complex
- Lists: list, tuple, range
- Folder (keys with a value, like a dictionary): dict
- Set (a list where values are not repeated): set, frozenset
- Boolean value (true or false): bool
- Binary data types: bytes, bytearray, memoryview
- Empty value: None
How is the data type defined?
Python can infer the data type itself. You can test this with the type function.
x = 3
print(type(x))
<class 'int'>
y = "3"
print(type(y))
<class 'str'>
Numbers
The most common data types for numbers are int and float. int (Integer) means an integer, while float (Floating point) means a decimal number.
a = 3
print(type(a))
<class 'int'>
b = 3.5
print(type(b))
<class 'float'>
Text
The data type of the text is str, which stands for String (string).
a = "hi"
print(type(a))
<class 'str'>
Truth value
The data type for True/False values is bool, which stands for Boolean.
a = True
print(type(a))
<class 'bool'>
b = False
print(type(b))
<class 'bool'>
Type coercion
Let's go back to the previous example. The code caused an error because the operands of the plus operator (left and right side) were not compatible, one was int and the other was str, which cannot be concatenated together.
a = 3
b = "5"
print(a + b)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'str'
We could solve the problem by forcing the number to text, or text to number. Let's try both.
The number is converted to text with the str function.
a = 3
b = "5"
print(str(a) + b)
35
The text is converted to a number using the int function.
a = 3
b = "5"
print(a + int(b))
8
Exercise
This is an example of how the program should function.
Enter the first number: 5
Enter another number: 10
15
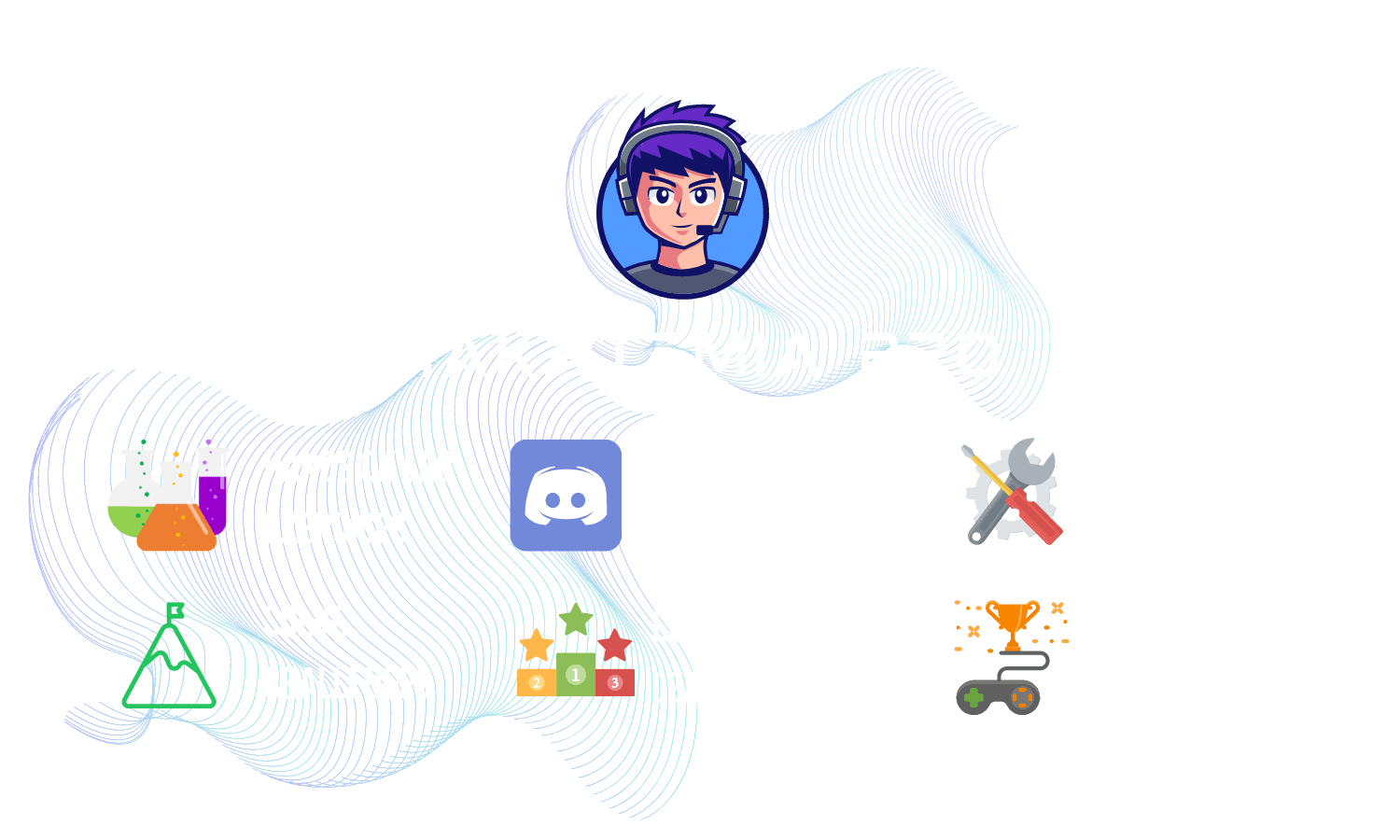
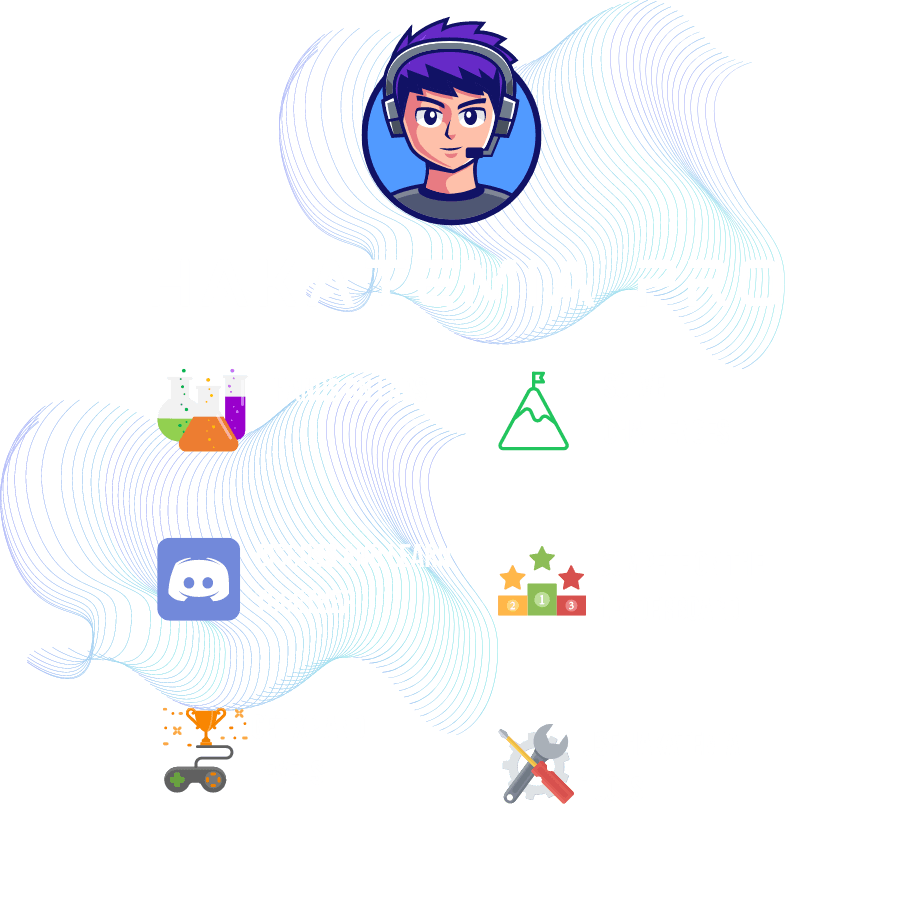
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.