What are functions?
Functions play an important role in Python programming. With a function, you can name a logical entity that performs a specific action. Arguments can be passed to a function to control its execution. The code inside a function is executed only when the function is called.
What functions are needed?
Functions are important because they help in reducing code repetition. If the same operation needs to be performed multiple times in a program, it is advisable to write that operation as a function, which can then be called at different points in the program. This reduces the amount of code to be written and makes the program easier to maintain.
Python functions
In Python, functions are defined using the def keyword, followed by the function name and any arguments. The function code is written indented on the following lines.
Example of a function that calculates the sum of two numbers:
def sum(a, b):
result = a + b
return result
In this function there are two arguments, a and b. Inside the function the sum of the arguments is calculated and saved in the variable result. Finally, the result is returned as the result of the function.
Function return values
Functions can return or not return values. For example, the function "tallenna_peli" may not return anything, it just saves the game. But the function "summa" (like the example above) is logically expected to return the summed total to the function caller.
Functions in Python return a value with the return keyword. After that, the execution of the function ends, and no more lines of code inside the function are executed. The return statement can also be made without any arguments, which terminates the function execution without a return value.
Calling a function
The function is called by giving the function name and arguments in parentheses. For example, the above function could be called as follows:
result = sum(2, 3)
print(result)
This would print 5, because the result returned by the function sum(2, 3) is saved in the variable result and then printed with the print function.
Exercise
Write a function named largest_number that accepts a list of numbers and returns the largest number. You can assume that the list contains at least one number.
An example of how the function should work:
Reading List = [3, 7, 1, 9, 4]
print(largest_number(numberlist)) # Prints 9
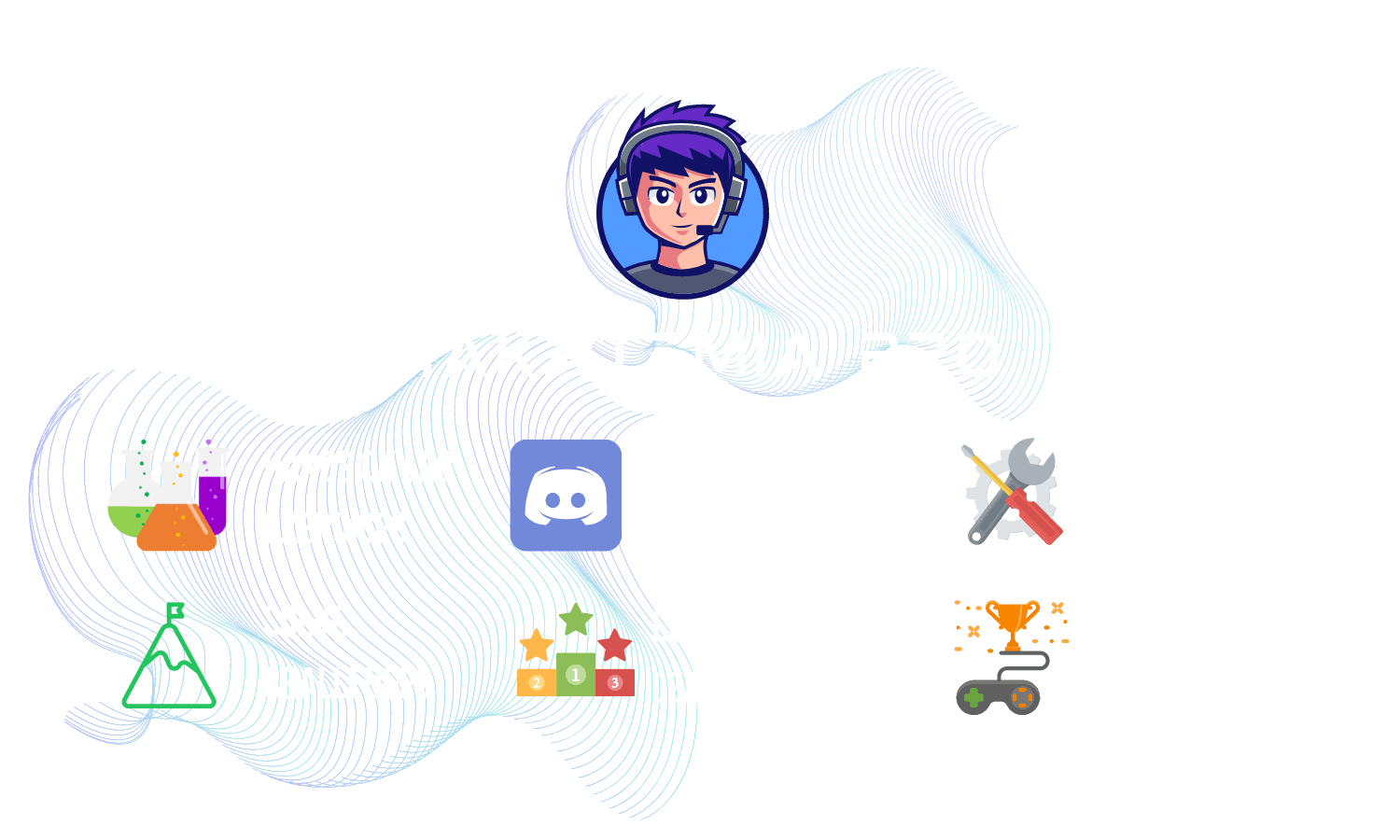
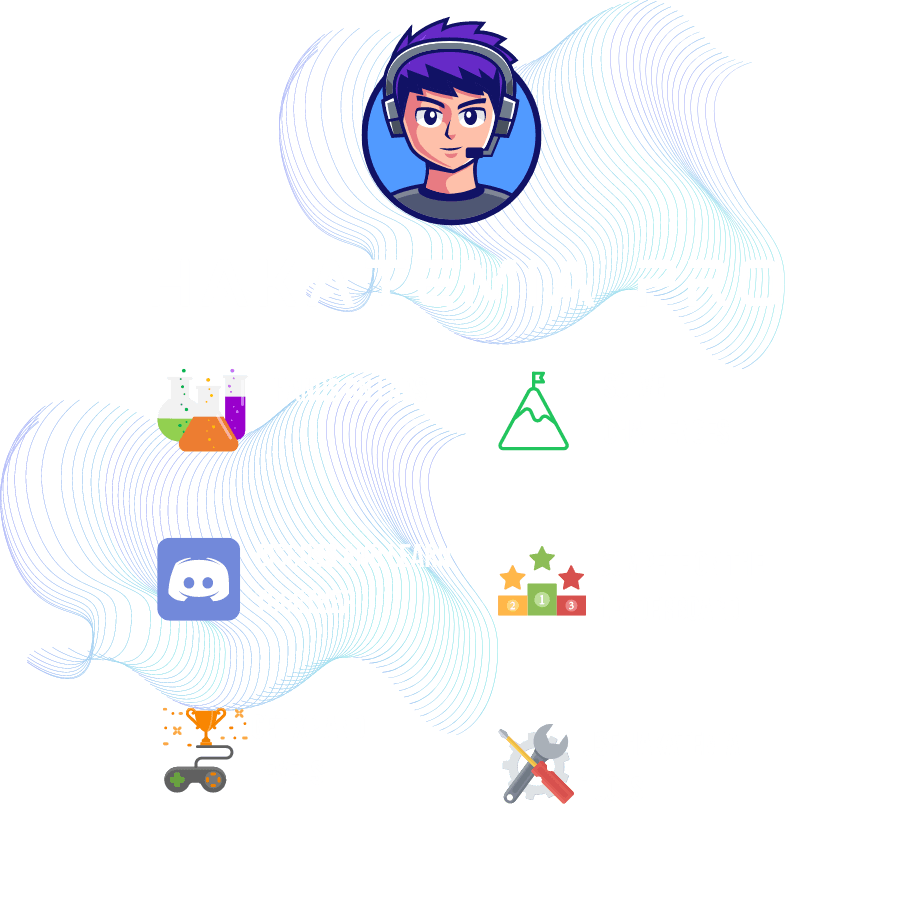
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.