What are imports?
When programming, it is advisable to use existing code as much as possible. Of course, the code must be of high quality and secure, so it is important to be careful about running code made by others on your own computer or in your own program.
In Python, however, there is fortunately a "standard library", i.e. a standard library of various modules for many different needs. The functionality of the modules is not directly available in your code, but they need to be imported separately into your program for use. And this is done with the import command.
Case study: Random numbers
Let's take an example, random numbers. Let's say we want to display a random number between zero and one hundred. We could perhaps build our own function that would do something like this:
- Take the computer time in nanoseconds.
- Retrieve the remaining disk space of the computer in bytes.
- Retrieve the computer's serial number.
- Connect all these, add a leap, divide with a flower and multiply the remainder with a stone.
Generating random numbers is such a common need that there is a module for it in Python's standard library: random. We can import the module into our program as follows:
import random
If we want to see what the module offers, we can do this with the dir command.
import random
print(dir(random))
['BPF', 'LOG4', 'NV_MAGICCONST', 'RECIP_BPF', 'Random', 'SG_MAGICCONST', 'SystemRandom', 'TWOPI', '_ONE', '_Sequence', '_Set', '__all__', ' __builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', '_accumulate', '_acos', '_bisect', '_ceil', '_cos' , '_e', '_exp', '_floor', '_index', '_inst', '_isfinite', '_log', '_os', '_pi', '_random', '_repeat', '_sha512', ' _sin', '_sqrt', '_test', '_test_generator', '_urandom', '_warn', 'betavariate', 'choice', 'choices', 'expovariate', 'gammavariate', 'gauss', 'getrandbits' , 'getstate', 'lognormvariate', 'normalvariate', 'paretovariate', 'randbytes', 'randint', 'random', 'randrange', 'sample', 'seed', 'setstate', 'shuffle', ' triangular', 'uniform', 'vonmisesvariate', 'weibullvariate']
However, it is easier to just consult the Python documentation: random.

If we want a random number between 1 and 10: we can call the randint function. And since the function is from the random module, it is called like this:
random.randint(1, 10)
The syntax is thus <MODULE><PERIOD><FUNCTION>(<PARAMETERS>). So for example:
print(random.randint(1, 10))
7
print(random.randint(1, 10))
2
Exercise
Example of how the program should function:
python3 app.py
Number of eyes on the dice: 3
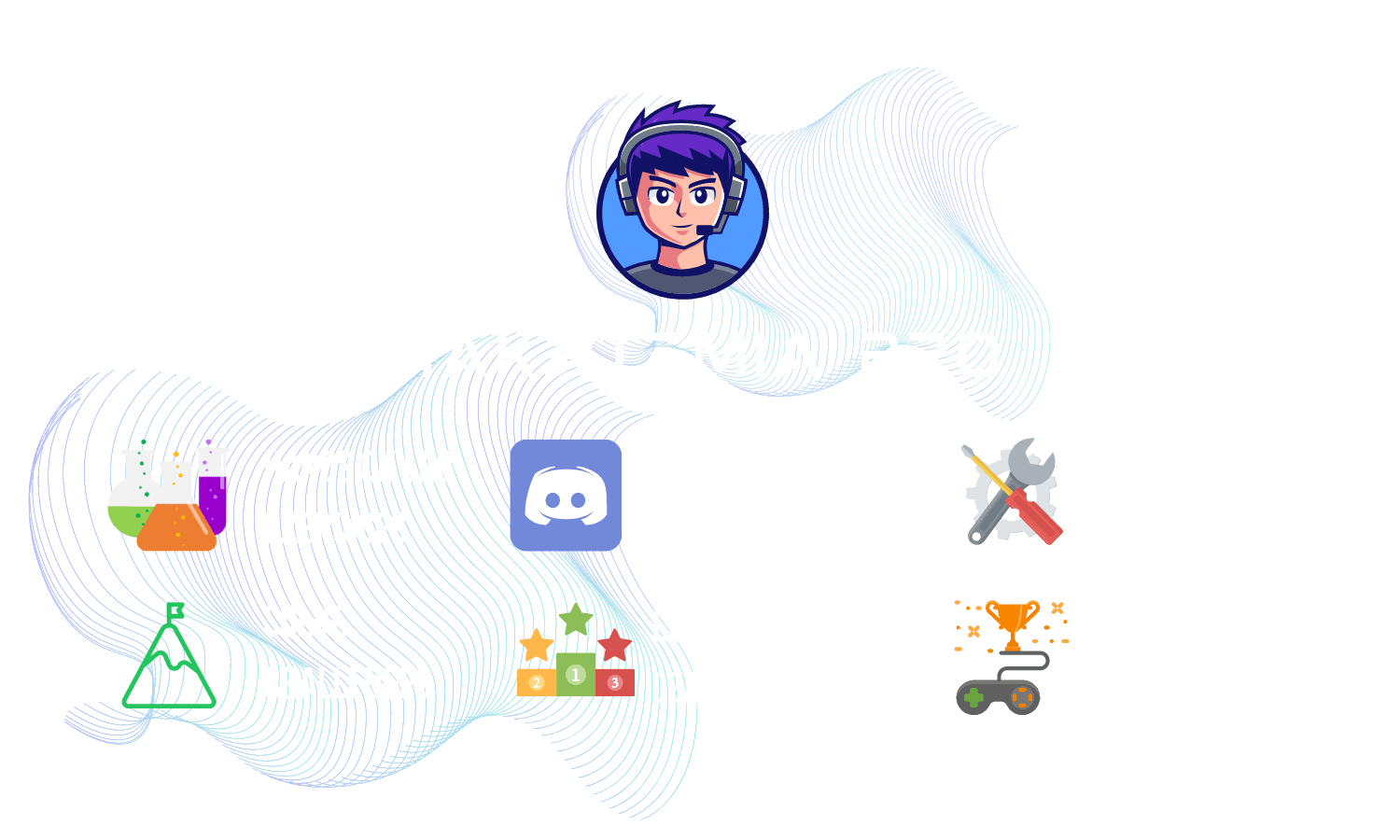
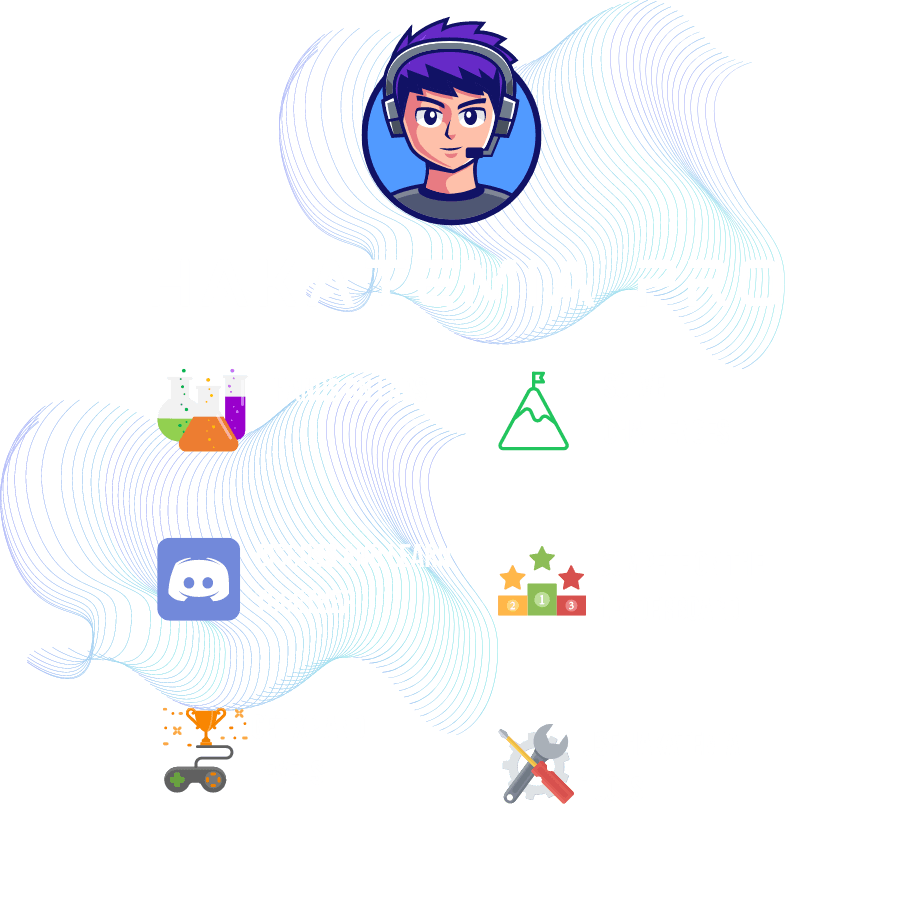
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.