What are arrays used for?
Let's say we have an application for creating a shopping list. We could do something like this:
purchase_1 = input("What is the first purchase?: ")
purchase_2 = input("What is the second purchase?: ")
purchase_3 = input("What is the third purchase?: ")
print("Shopping list: ", purchase_1, purchase_2, purchase_3)
python3 ./app.py
What's the first purchase?: Ketchup
What's the second purchase?: Cucumber
What is the third purchase?: Tomato
Shopping list: Ketchup Cucumber Tomato
The program works, but it has quite a few limitations. The most significant one is that there can be exactly three purchases, no more and no less. We can solve this problem with lists.
Lists
List is an array data type in Python, and its syntax is brackets, with the list elements inside (such as shopping items on a shopping list).
shopping_list = ["Cucumber", "Ketchup", "Tomato"]
print(shopping_list)
['Cucumber', 'Ketchup', 'Tomato']
The list includes useful functions, such as append, clear, count, index, reverse, and sort.
print(dir(shopping_list))
[... 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
Adding an item to the list
Elements can be added to the end of the list with the append function.
shopping_list.append("Basilika")
print(shopping_list)
['Cucumber', 'Ketchup', 'Tomato', 'Basil']
Retrieving an element (indexing) from the list
You can for example print a specific value from a list with its index. In Python, indices start from zero, so the index of the first element is 0, the next one is 1, etc.
third_purchase = shopping_list[2]
print(third_purchase)
Tomato
Removing an item from the list
You can remove an item from the list by using the pop function with its index.
shopping_list.pop(1)
print(shopping_list)
['Cucumber', 'Tomato', 'Basil']
Alternatively, you can remove the first item from the list that has a certain value. This can be done with the remove function.
shopping_list.remove('Cucumber')
print(shopping_list)
['Tomato', 'Basil']
List sorting
The list can be sorted using the sort function. By default, the sorting is done in alphabetical/numerical order.
numbers = [5, 2, 4, 3, 8, 2]
numbers.sort()
print(numbers)
[2, 2, 3, 4, 5, 8]
texts = ['Piano', 'Monkey', 'Mackerel']
texts.sort()
print(texts)
['Monkey', 'Mackerel', 'Piano']
Translation of the List
The list can be reversed with the reverse function.
numbers = [5, 2, 4, 3, 8, 2]
numbers.sort()
print(numbers)
[2, 2, 3, 4, 5, 8]
numbers.reverse()
print(numbers)
[8, 5, 4, 3, 2, 2]
Searching for an element in a list
Sometimes you want to know from which index a specific element is found. You can do this with the index function. For example, "Tomaatti" is found at index 2 (remember that indices start from zero).
shopping_list = ["Cucumber", "Ketchup", "Tomato"]
print(shopping_list.index("Tomato"))
2
List length
The length of the list, that is, the number of elements in the list, is obtained using the built-in len function.
shopping_list = ["Cucumber", "Ketchup", "Tomato", "Avocado", "Pasta", "Chili"]
print(len(shopping_list))
6
List subset (subset)
The list subset, or subset, is obtained with the following syntax.
LIST[FROM_INDEX:TO_INDEX + 1]
For example, a subset of the list starting from index 1 (which is Ketchup) and ending at index 3 (Avocado) would be taken as follows.
shopping_list = ["Cucumber", "Ketchup", "Tomato", "Avocado", "Pasta", "Chili"]
subset = shopping_list[1:4]
print(subset)
['Ketchup', 'Tomato', 'Avocado']
Exercise
Example of how the program should work:
python3 ./app.py
Enter the first number: 4
Enter another number: 17
Enter the third number: 1
[1, 4, 17]
Be careful with data types. If you don't convert user-read values to int type (with typecasting), the program won't sort numbers correctly because as a string (str type), for example, 17 would come before 4 in alphabetical order.
If you don't remember how to read user input as a number, take a look at previous modules.
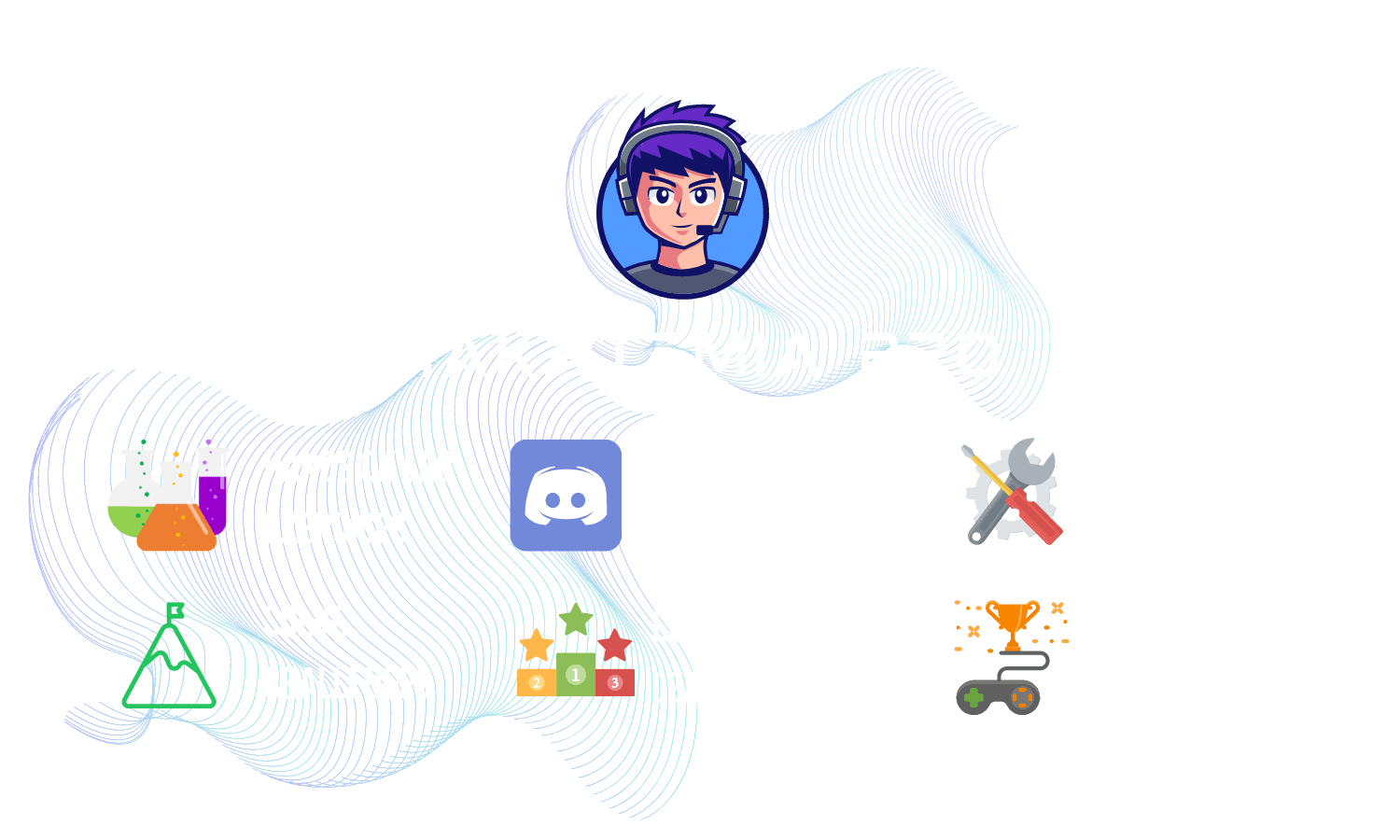
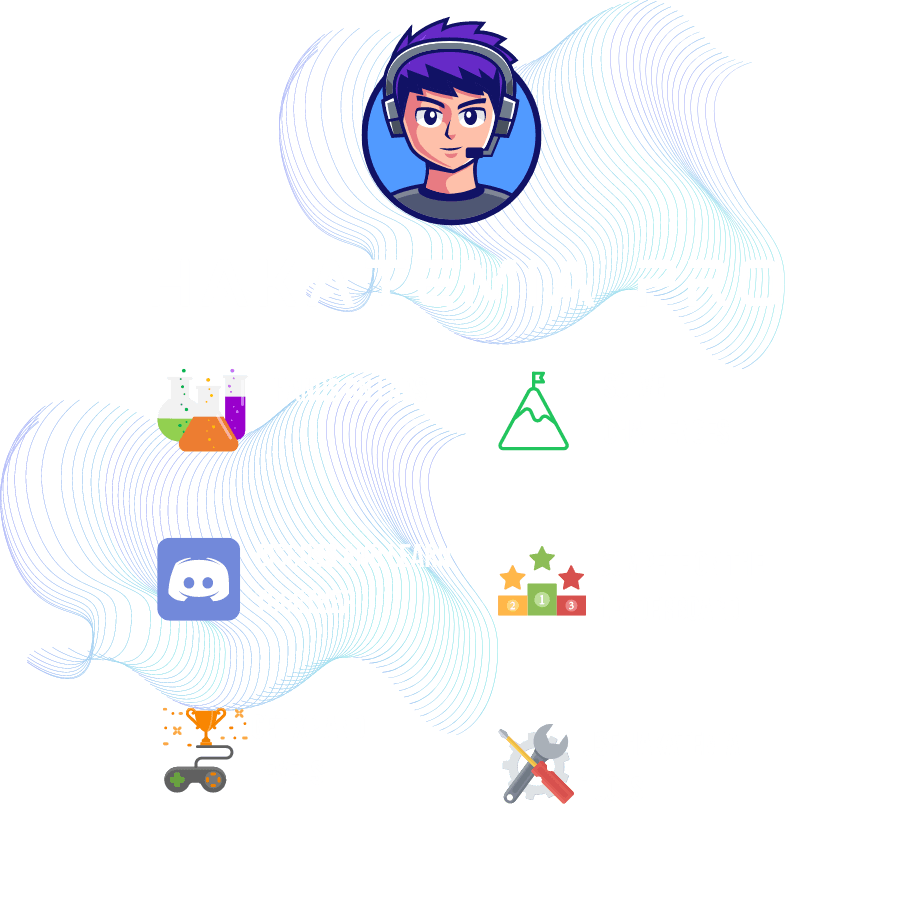
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.