So far, we have familiarized ourselves with two different SQL injection vulnerabilities, one of which was the SELECT statement in the login screen and the other was the SELECT statement on the credit card page. However, the vulnerability may exist in any type of SQL query, and in this module, we will examine the injection that occurs in the INSERT statement.
What is an INSERT statement?
When data is retrieved using the SELECT statement, data is added to the database using the INSERT statement.
The format of the INSERT statement is simple:
INSERT INTO <table name> (column names) VALUES (column values)
Let's say, for example, that we have an online banking application that records a completed transaction in its database. In this case, the application could write the columns amount, recipient's IBAN, sender's IBAN, reference number, and message into a table named transaction, and then provide the values as follows:
INSERT INTO transaction (amount, recipient_iban, sender_iban, reference, message) VALUES (25, 'SD31RAYV75830132022610', 'AQ07SXPO99612579131812', '123', 'test')
Alternatively, if the application would like to add two rows at a time to the database, it could be done as follows:
INSERT INTO transaction (amount, recipient_iban, sender_iban, reference, message) VALUES (25, 'SD31RAYV75830132022610', 'AQ07SXPO99612579131812', '123', 'test'),(100, 'SD31RAYV75830132022610', 'AQ07SXPO996125 79131812', '124', 'test 2')
Injection in the INSERT Statement
If there is injection in the application, let's say, in the reference number field, we could for example:
- Escape out of the reference number text.
- Add the message ('test') yourself.
- Add VALUES parentheses yourself.
- Comment out the rest of the query.
', 'test')--
In this case, the application can be run the exact same query as it would normally, with the difference that now we control part of the SQL. We can replace the text 'test' with something else, for example, the database version:
',@@version)--
At this point, the application runs the following query and the bank transfer should appear in the application, where the message field will display the database version.
INSERT INTO transaction (amount, recipient_iban, sender_iban, reference, message) VALUES (25, 'SD31RAYV75830132022610', 'AQ07SXPO99612579131812', '123', @@version)-- ', 'test')

Subqueries
Now we just need to retrieve something more useful from the application, such as the email address and password of the admin user, instead of the version of the database. In this case, it can be done with a subquery, which means a query embedded inside another query.
By substituting @@version with a subquery that returns the email address and password from a user whose admin column value is True, you can pass this exercise.

Limiting the results of a subquery to one using the LIMIT clause
Note the use of the LIMIT clause. LIMIT restricts the subquery search results to one. Otherwise, the SQL query would crash if there were multiple users with a admin column value of True.
What if the injection is in the last part of the INSERT statement?
If the injection is in the last part of the VALUES, in this case the message field, of course there is no next field to attack. In this case, a solution could be to add a completely new set of values after VALUES, in which case the query would only create one harmless row in the database, and another row where you can freely inject whatever you want.
VALUES(first values),(following values)
MySQLi INSERT Injection
In this laboratory, you can practice injection into the INSERT statement by adding subqueries to the values of the VALUES statement. You will also learn how to use the LIMIT statement to limit the number of rows returned by the subquery.
Objective
Login as an admin user.
Exercises
Flag
Find the flag from the lab environment and enter it below.
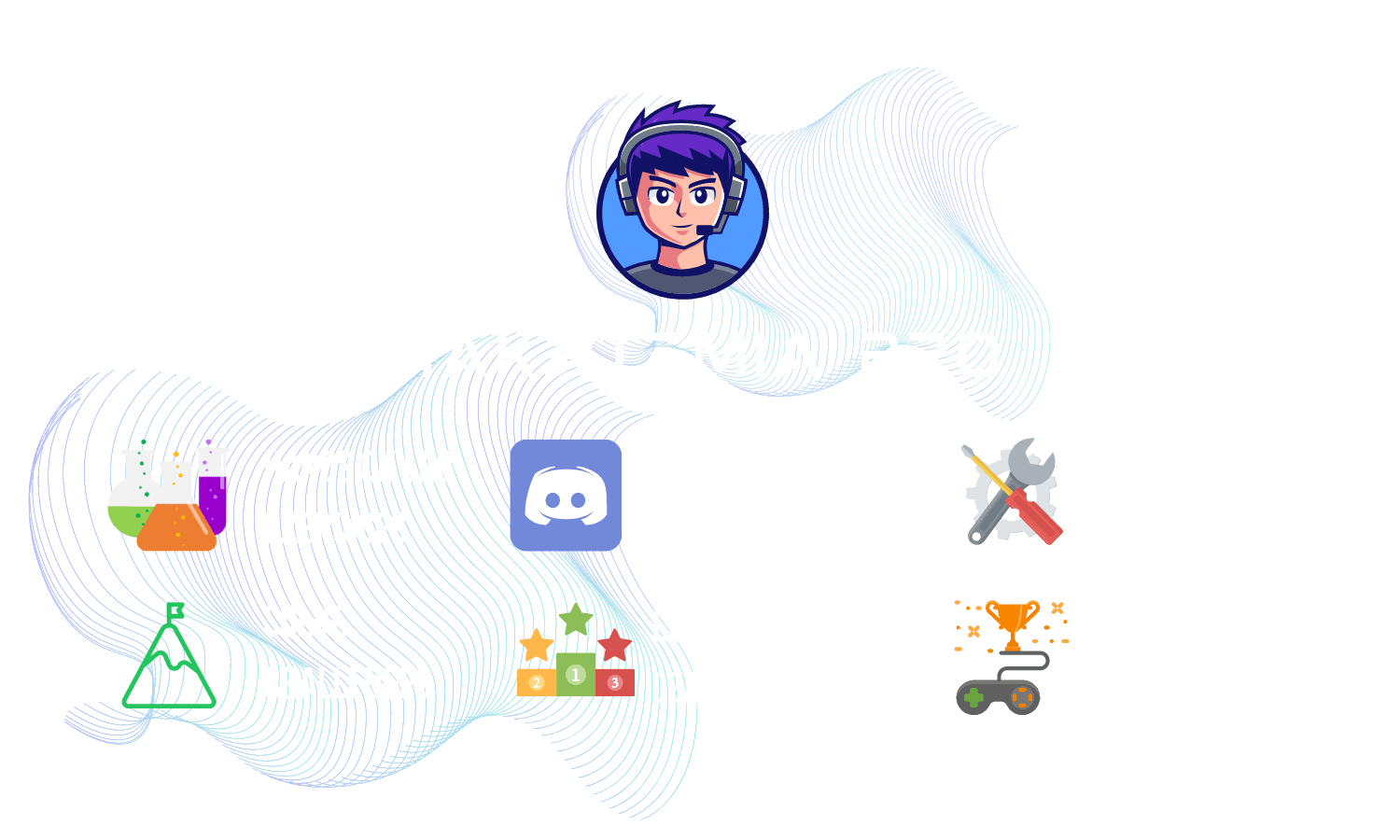
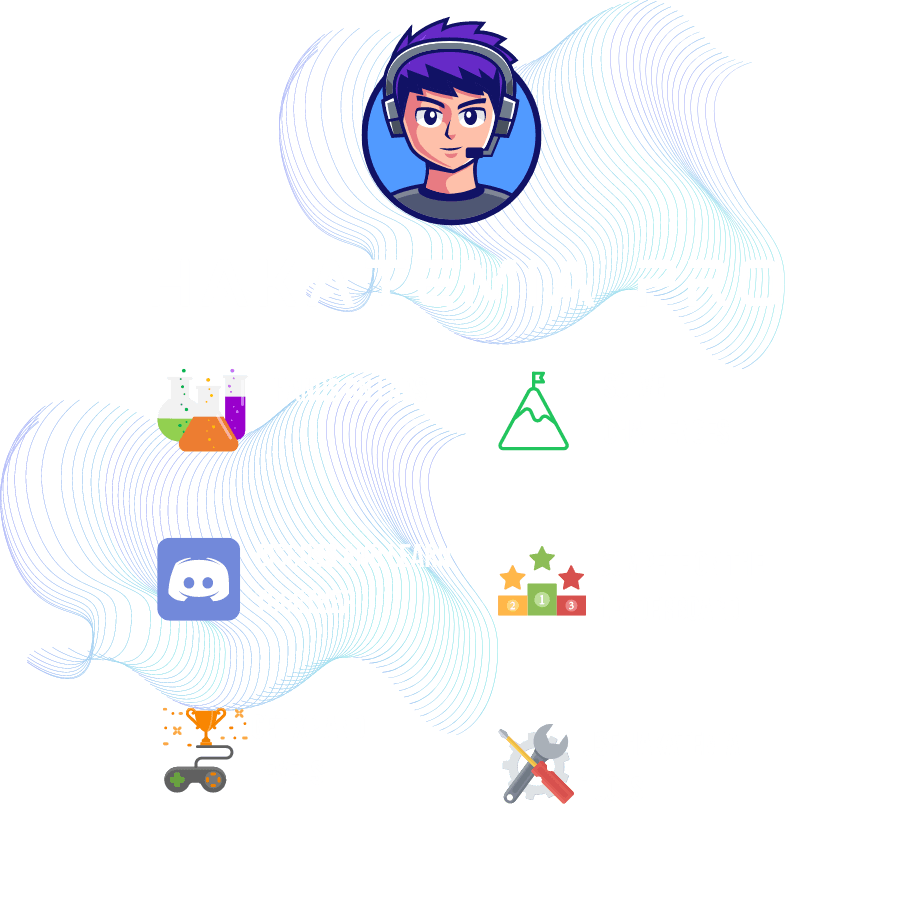
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.